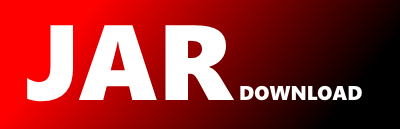
eu.limetri.ygg.client.YggClient Maven / Gradle / Ivy
package eu.limetri.ygg.client;
import eu.limetri.ygg.api.BusinessProcessRegistry;
import eu.limetri.ygg.api.BusinessProcessTemplateRegistry;
import eu.limetri.ygg.api.Capability;
import eu.limetri.ygg.api.CapabilityList;
import eu.limetri.ygg.api.CapabilityRegistry;
import eu.limetri.ygg.api.CapabilityType;
import eu.limetri.ygg.api.CapabilityTypeRegistry;
import eu.limetri.ygg.api.NameTranslator;
import eu.limetri.ygg.api.RegistryException;
import eu.limetri.ygg.api.RequestMessage;
import eu.limetri.ygg.api.ResponseMessage;
import javax.ws.rs.RedirectionException;
import javax.ws.rs.client.Entity;
import javax.ws.rs.core.MediaType;
import static javax.ws.rs.core.MediaType.APPLICATION_JSON_TYPE;
import static javax.ws.rs.core.MediaType.APPLICATION_XML_TYPE;
import javax.ws.rs.core.Response;
import static javax.ws.rs.core.Response.Status.NO_CONTENT;
import static javax.ws.rs.core.Response.Status.SEE_OTHER;
import org.jboss.resteasy.client.jaxrs.ResteasyClient;
import org.jboss.resteasy.client.jaxrs.ResteasyClientBuilder;
import org.jboss.resteasy.client.jaxrs.ResteasyWebTarget;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* Copyright (C) 2008-2013 LimeTri. All rights reserved.
*
* This library is free software: you can redistribute it and/or modify it under
* the terms of the GNU General Public License as published by the Free Software
* Foundation, either version 3 of the License, or (at your option) any later
* version.
*
* There are special exceptions to the terms and conditions of the GPLv3 as it
* is applied to this software, see the FLOSS License Exception
* .
*
* This library is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU General Public License for more
* details.
*
* You should have received a copy of the GNU General Public License along with
* this library. If not, see .
*/
/**
* To use this client make sure there is a META-INF/keycloak.json available on
* the classpath. This keycloak config file should point to a oauth client with
* the appropriate grants to the ygg server application. The oauth client should
* be able to access username, email etc from the token.
*
* Before using the functional methods of this client use {@link YggClient#authenticate()
* }. authenticate will initiate a browser login if needed.
*
* This client is suited for use in installed applications.
*
*
* @author Timon Veenstra
*/
public abstract class YggClient {
private final String baseUrl;
private static final Logger log = LoggerFactory.getLogger(YggClient.class);
public YggClient(String baseUrl) {
this.baseUrl = baseUrl;
}
public abstract void authenticate() throws AuthenticationException;
public abstract String getTokenString() throws AuthenticationException;
private static final MediaType DEFAULT_MEDIA_TYPE = APPLICATION_XML_TYPE;
private MediaType mediaType = DEFAULT_MEDIA_TYPE;
/**
* Sets preferred media type to use in communication to Ygg server. Choose
* either one of:
*
* - {@link APPLICATION_XML_TYPE}
* - {@link APPLICATION_JSON_TYPE}
*
*
*
* @param mediaType
*/
public void setMediaype(MediaType mediaType) {
if (!APPLICATION_XML_TYPE.equals(mediaType) && !APPLICATION_JSON_TYPE.equals(mediaType)) {
throw new IllegalArgumentException("unsupported MediaType " + mediaType + " use either APPLICATION_XML_TYPE or APPLICATION_JSON_TYPE");
}
this.mediaType = mediaType;
}
/**
* Client side proxy implementation of {@link CapabilityRegistry}
*
* @return
* @throws eu.limetri.ygg.client.AuthenticationException
*/
public CapabilityRegistry getCapabilityRegistry() throws AuthenticationException {
// Client client = ClientBuilder.newBuilder().register(new VoidReader()).build();
// WebTarget target = client.target(this.baseUrl);
// return WebResourceFactory.newResource(CapabilityRegistry.class, target);
ResteasyClient client = new ResteasyClientBuilder().build();
client.register(new AuthHeadersRequestFilter(getTokenString()));
ResteasyWebTarget target = client.target(this.baseUrl);
return target.proxyBuilder(CapabilityRegistry.class)
.defaultConsumes(mediaType)
.defaultProduces(mediaType)
.build();
}
/**
* Client side proxy implementation of {@link CapabilityRegistry}
*
* @return
* @throws eu.limetri.ygg.client.AuthenticationException
*/
public CapabilityTypeRegistry getCapabilityTypeRegistry() throws AuthenticationException {
// Client client = ClientBuilder.newBuilder().register(new VoidReader()).build();
// WebTarget target = client.target(this.baseUrl);
// return WebResourceFactory.newResource(CapabilityTypeRegistry.class, target);
ResteasyClient client = new ResteasyClientBuilder().build();
client.register(new AuthHeadersRequestFilter(getTokenString()));
ResteasyWebTarget target = client.target(this.baseUrl);
return target.proxyBuilder(CapabilityTypeRegistry.class)
.defaultConsumes(mediaType)
.defaultProduces(mediaType)
.build();
}
/**
* client side proxy implementation of {@link BusinessProcessRegistry}
*
* @return
* @throws eu.limetri.ygg.client.AuthenticationException
*/
public BusinessProcessRegistry getBusinessProcessRegistry() throws AuthenticationException {
// Client client = ClientBuilder.newBuilder().register(new VoidReader()).build();
// WebTarget target = client.target(this.baseUrl);
// return WebResourceFactory.newResource(BusinessProcessRegistry.class, target);
ResteasyClient client = new ResteasyClientBuilder().build();
client.register(new AuthHeadersRequestFilter(getTokenString()));
ResteasyWebTarget target = client.target(this.baseUrl);
return target.proxyBuilder(BusinessProcessRegistry.class)
.defaultConsumes(mediaType)
.defaultProduces(mediaType)
.build();
}
/**
* client side proxy implementation of
* {@link BusinessProcessTemplateRegistry}
*
* @return
* @throws eu.limetri.ygg.client.AuthenticationException
*/
public BusinessProcessTemplateRegistry getBusinessProcessTemplateRegistry() throws AuthenticationException {
// Client client = ClientBuilder.newBuilder().register(new VoidReader()).build();
// WebTarget target = client.target(this.baseUrl);
// return WebResourceFactory.newResource(BusinessProcessRegistry.class, target);
ResteasyClient client = new ResteasyClientBuilder().build();
client.register(new AuthHeadersRequestFilter(getTokenString()));
ResteasyWebTarget target = client.target(this.baseUrl);
return target.proxyBuilder(BusinessProcessTemplateRegistry.class)
.defaultConsumes(mediaType)
.defaultProduces(mediaType)
.build();
}
/**
* Returns all available capabilities
*
* @return
* @throws eu.limetri.ygg.client.AuthenticationException
*/
public CapabilityList getCapabilities() throws AuthenticationException {
try {
return getCapabilityRegistry().getCapabilities();
} catch (RedirectionException e) {
log.error(e.getMessage());
//
// when the authentication token is not correct, the server will try to redirect to
// login page when configured as being public. When configured as beared-only it will
// try to redirect to "Bearer-only applications are not allowed to initiate browser login"
// page, so we can handle both the same way
//
throw new AuthenticationException("Invalid authentication token");
}
}
/**
* use a capability
*
* @param capability
* @param requestMessage, subclass defined in capability type definition
* @return
* @throws eu.limetri.ygg.client.AuthenticationException
*/
public ResponseMessage use(Capability capability, RequestMessage requestMessage) throws AuthenticationException {
CapabilityType capabilityType = getCapabilityTypeRegistry().getCapabilityType(capability.getCteId());
// Client client = ClientBuilder.newBuilder().register(new VoidReader()).build();
log.debug("initializing web target with base url {}", this.baseUrl);
// WebTarget target = client.target(this.baseUrl);
// CapabilityUsage resource = WebResourceFactory.newResource(CapabilityUsage.class, target);
ResteasyClient client = new ResteasyClientBuilder().build();
client.register(new AuthHeadersRequestFilter(getTokenString()));
ResteasyWebTarget target = client.target(this.baseUrl);
log.debug("invoking resource proxy with name {} and id {}", NameTranslator.translateForURI(capabilityType), capability.getId());
Response response = target
.path(CapabilityRegistry.RESOURCE_CAPABILITIES)
.path(NameTranslator.translateForURI(capabilityType))
.path(CapabilityRegistry.RESOURCE_USE)
.request()
.post(Entity.entity(requestMessage, mediaType));
switch (Response.Status.fromStatusCode(response.getStatus())) {
case NO_CONTENT:
log.debug("Response.Status.fromStatusCode:NO_CONTENT");
break;
case SEE_OTHER:
log.debug("Response.Status.fromStatusCode:SEE_OTHER");
ResteasyWebTarget redirect = client.target(response.getHeaderString("Location"));
log.debug("following redirect");
response = redirect
.request()
.post(Entity.entity(requestMessage, mediaType));
break;
case ACCEPTED:
log.debug("Response.Status.fromStatusCode:accepted");
break;
default:
log.debug("unexpected respoonse: {}",Response.Status.fromStatusCode(response.getStatus()));
throw new RegistryException(response.readEntity(String.class),RegistryException.Type.UNKNOWN_TECHNICAL);
}
return response.readEntity(ResponseMessage.class);
}
// CapabilityUsage resource = target.proxyBuilder(CapabilityUsage.class)
// .defaultConsumes(DEFAULT_MEDIA_TYPE)
// .defaultProduces(DEFAULT_MEDIA_TYPE)
// .build();
//
//
// log.debug("invoking resource proxy with name {} and id {}", NameTranslator.translateForURI(capabilityType), capability.getId());
// return resource.use(capability.getId(), NameTranslator.translateForURI(capabilityType), requestMessage);
// ResponseMessage responseMessage = null;
// try {
//
//
//
//
// ResteasyClient client = new ResteasyClientBuilder().build();
//
// ResteasyWebTarget target = client
// .target("http://localhost:8080/capabilities/1/use");
//
// Response response = target.request().post(
// Entity.entity(requestMessage, MediaType.APPLICATION_XML));
//
// switch(Response.Status.fromStatusCode(response.getStatus())){
// case NO_CONTENT:break;
// case SEE_OTHER:break;
// default: break;
// }
//
// if (response.getStatus() != 200) {
// throw new RuntimeException("Failed : HTTP error code : "
// + response.getStatus());
// }
//
// System.out.println("Server response : \n");
// System.out.println(response.readEntity(String.class));
//
// response.close();
//
// } catch (Exception e) {
//
// e.printStackTrace();
//
// }
//
// return responseMessage;
// }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy