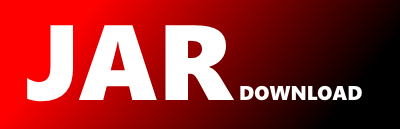
eu.limetri.ygg.client.YggClient.orig Maven / Gradle / Ivy
package eu.limetri.ygg.client;
import eu.limetri.ygg.api.BusinessProcessRegistry;
import eu.limetri.ygg.api.Capability;
import eu.limetri.ygg.api.CapabilityList;
import eu.limetri.ygg.api.CapabilityRegistry;
import eu.limetri.ygg.api.CapabilityType;
import eu.limetri.ygg.api.CapabilityTypeRegistry;
import eu.limetri.ygg.api.CapabilityUsage;
import eu.limetri.ygg.api.NameTranslator;
import eu.limetri.ygg.api.RequestMessage;
import eu.limetri.ygg.api.ResponseMessage;
import java.util.ArrayList;
import javax.ws.rs.client.Client;
import javax.ws.rs.client.ClientBuilder;
import javax.ws.rs.client.WebTarget;
import javax.ws.rs.core.Cookie;
import javax.ws.rs.core.Form;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.MultivaluedHashMap;
import javax.ws.rs.core.MultivaluedMap;
import org.glassfish.jersey.client.proxy.WebResourceFactory;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* Copyright (C) 2008-2013 LimeTri. All rights reserved.
*
* This library is free software: you can redistribute it and/or modify it under
* the terms of the GNU General Public License as published by the Free Software
* Foundation, either version 3 of the License, or (at your option) any later
* version.
*
* There are special exceptions to the terms and conditions of the GPLv3 as it
* is applied to this software, see the FLOSS License Exception
* .
*
* This library is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU General Public License for more
* details.
*
* You should have received a copy of the GNU General Public License along with
* this library. If not, see .
*/
/**
*
* @author Timon Veenstra
*/
public class YggClient {
private final String baseUrl;
private static final Logger log = LoggerFactory.getLogger(YggClient.class);
private static final MultivaluedMap headers = new MultivaluedHashMap();
static {
headers.add("Accept", MediaType.APPLICATION_XML);
}
public YggClient(String baseUrl) {
this.baseUrl = baseUrl;
}
/**
* Client side proxy implementation of {@link CapabilityRegistry}
*
* @return
*/
public CapabilityRegistry getCapabilityRegistry() {
Client client = ClientBuilder.newBuilder().register(new VoidReader()).build();
WebTarget target = client.target(this.baseUrl);
return WebResourceFactory.newResource(CapabilityRegistry.class, target, false, headers, new ArrayList(), new Form());
}
/**
* Client side proxy implementation of {@link CapabilityRegistry}
*
* @return
*/
public CapabilityTypeRegistry getCapabilityTypeRegistry() {
Client client = ClientBuilder.newBuilder().register(new VoidReader()).build();
WebTarget target = client.target(this.baseUrl);
return WebResourceFactory.newResource(CapabilityTypeRegistry.class, target, false, headers, new ArrayList(), new Form());
}
/**
* client side proxy implementation of {@link BusinessProcessRegistry}
*
* @return
*/
public BusinessProcessRegistry getBusinessProcessRegistry() {
Client client = ClientBuilder.newBuilder().register(new VoidReader()).build();
WebTarget target = client.target(this.baseUrl);
return WebResourceFactory.newResource(BusinessProcessRegistry.class, target, false, headers, new ArrayList(), new Form());
}
/**
* Returns all available capabilities
*
* @return
*/
public CapabilityList getCapabilities() {
return getCapabilityRegistry().getCapabilities();
}
/**
* use a capability
*
* @param capability
* @param requestMessage, subclass defined in capability type definition
* @return
*/
public ResponseMessage use(Capability capability, RequestMessage requestMessage) {
if (requestMessage.getCapabilityId() == 0) {
requestMessage.setCapabilityId(capability.getId());
log.warn("capability id in request message was not set, id from capability ({}) was set in the request message", capability.getId());
}
CapabilityType capabilityType = getCapabilityTypeRegistry().getCapabilityType(capability.getCteId());
Client client = ClientBuilder.newBuilder().register(new VoidReader()).build();
log.debug("initializing web target with base url {}", this.baseUrl);
WebTarget target = client.target(this.baseUrl);
CapabilityUsage resource = WebResourceFactory.newResource(CapabilityUsage.class, target, false, headers, new ArrayList(), new Form());
log.debug("invoking resource proxy with name {} and id {}", NameTranslator.translateForURI(capabilityType), capability.getId());
return resource.use(capability.getId(), NameTranslator.translateForURI(capabilityType), requestMessage);
// ResponseMessage responseMessage = null;
// try {
//
//
//
//
// ResteasyClient client = new ResteasyClientBuilder().build();
//
// ResteasyWebTarget target = client
// .target("http://localhost:8080/capabilities/1/use");
//
// Response response = target.request().post(
// Entity.entity(requestMessage, MediaType.APPLICATION_XML));
//
// switch(Response.Status.fromStatusCode(response.getStatus())){
// case NO_CONTENT:break;
// case SEE_OTHER:break;
// default: break;
// }
//
// if (response.getStatus() != 200) {
// throw new RuntimeException("Failed : HTTP error code : "
// + response.getStatus());
// }
//
// System.out.println("Server response : \n");
// System.out.println(response.readEntity(String.class));
//
// response.close();
//
// } catch (Exception e) {
//
// e.printStackTrace();
//
// }
//
// return responseMessage;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy