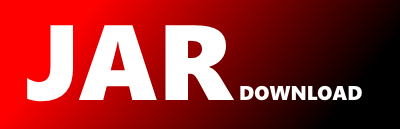
eu.lucaventuri.collections.ClassifiedMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fibry Show documentation
Show all versions of fibry Show documentation
The first Java Actor System supporting fibers from Project Loom
package eu.lucaventuri.collections;
import java.util.concurrent.ConcurrentHashMap;
import java.util.function.Function;
import java.util.function.Predicate;
/**
* This object contains a map grouped by class and a list; so it can be accessed in both ways.
* This object in intended to be used to filter messages based on their class and on some other attribute
*/
public class ClassifiedMap {
private final NodeLinkedList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy