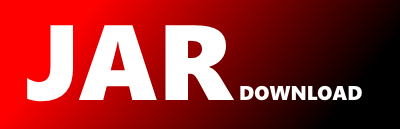
eu.lucaventuri.examples.ReceivingExample Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fibry Show documentation
Show all versions of fibry Show documentation
The first Java Actor System supporting fibers from Project Loom
package eu.lucaventuri.examples;
import eu.lucaventuri.fibry.MessageReceiver;
import eu.lucaventuri.fibry.ActorSystem;
import eu.lucaventuri.fibry.ReceivingActor;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ExecutionException;
import java.util.function.BiFunction;
public class ReceivingExample {
public static void main(String[] args) throws ExecutionException, InterruptedException {
BiFunction, Object, String> actorLogicReturn = (rec, msg) -> {
if (msg instanceof Integer) {
int n = ((Integer) msg).intValue();
String lookFor = "name" + n + ":";
String name = rec.receive(String.class, s -> s.startsWith(lookFor));
if (name == null)
return "NoName for " + n;
return "Name of " + n + ": " + name.substring(lookFor.length());
} else {
System.out.println("Skipping " + msg);
return "Skipping " + msg;
}
};
ReceivingActor
© 2015 - 2025 Weber Informatics LLC | Privacy Policy