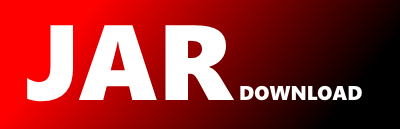
eu.lucaventuri.fibry.fsm.FsmBuilderBase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fibry Show documentation
Show all versions of fibry Show documentation
The first Java Actor System supporting fibers from Project Loom
package eu.lucaventuri.fibry.fsm;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.function.Consumer;
class StateData>, I> {
final C consumer;
final List> transtions = new ArrayList();
StateData(C consumer) {
this.consumer = consumer;
}
}
/**
* Builder that can create a Fsm, Finite State machine
*
* @param Type of the states, from an enum
* @param Type of the messages (they will need to support equals)
*/
abstract class FsmBuilderBase>, I> {
final Map> mapStatesEnum = new HashMap<>();
public class InState {
final List> transitions;
public InState(List> transitions) {
this.transitions = transitions;
}
public FsmBuilderBase.InState goTo(S targetState, M message) {
transitions.add(new TransitionEnum<>(message, targetState));
return this;
}
public InState addState(S state, C consumer) {
return FsmBuilderBase.this.addState(state, consumer);
}
public FsmTemplate>, I> build() {
return FsmBuilderBase.this.build();
}
}
public InState addState(S state, C consumer) {
if (mapStatesEnum.containsKey(state))
throw new IllegalArgumentException("State " + state + "already defined!");
mapStatesEnum.putIfAbsent(state, new StateData(consumer));
return new InState(mapStatesEnum.get(state).transtions);
}
public FsmTemplate build() {
return new FsmTemplate<>(mapStatesEnum);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy