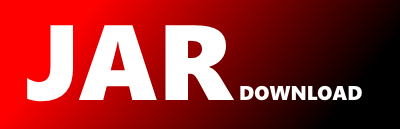
eu.lucaventuri.collections.ConstrainedMapLRU Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fibry Show documentation
Show all versions of fibry Show documentation
The first Java Actor System supporting fibers from Project Loom
package eu.lucaventuri.collections;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.function.Consumer;
/**
* Thread safe map that cannot grow past a certain size. Entries are evicted in a LRU order.
* Access is synchronized on all the operations
*/
public class ConstrainedMapLRU {
private final Map map;
private final int maxSize;
/**
* Constructor
*
* @param maxSize Maximum size allowed for the map
*/
public ConstrainedMapLRU(int maxSize, Consumer> onRemoval) {
map = new LinkedHashMap<>(maxSize, 0.75f, true) {
protected boolean removeEldestEntry(Map.Entry eldest) {
boolean toBeRemoved = size() > maxSize;
if (onRemoval != null && toBeRemoved)
onRemoval.accept(eldest);
return toBeRemoved;
}
};
this.maxSize = maxSize;
}
public synchronized V get(K key) {
return map.get(key);
}
public synchronized V put(K key, V value) {
assert map.size() <= maxSize;
V oldValue = map.put(key, value);
assert map.size() <= maxSize;
return oldValue;
}
public synchronized V remove(K key) {
return map.remove(key);
}
public synchronized int size() {
return map.size();
}
public synchronized void clear() {
map.clear();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy