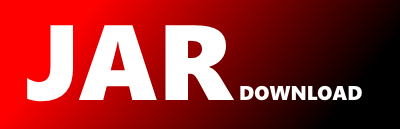
eu.lucaventuri.examples.ActorInheritanceExample Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fibry Show documentation
Show all versions of fibry Show documentation
The first Java Actor System supporting fibers from Project Loom
package eu.lucaventuri.examples;
import eu.lucaventuri.common.SystemUtils;
import eu.lucaventuri.fibry.CreationStrategy;
import eu.lucaventuri.fibry.CustomActor;
import eu.lucaventuri.fibry.CustomActorWithResult;
import eu.lucaventuri.fibry.FibryQueue;
import javax.sound.midi.SoundbankResource;
import java.util.concurrent.ExecutionException;
class ActorInherit extends CustomActor
© 2015 - 2025 Weber Informatics LLC | Privacy Policy