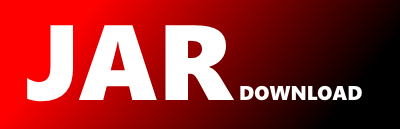
eu.lucaventuri.fibry.MiniQueue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fibry Show documentation
Show all versions of fibry Show documentation
The first Java Actor System supporting fibers from Project Loom
package eu.lucaventuri.fibry;
import java.util.concurrent.LinkedBlockingDeque;
import java.util.concurrent.TimeUnit;
import eu.lucaventuri.collections.PriorityMiniQueue;
public interface MiniQueue {
boolean add (T message);
default boolean offer (T message) {
try {
return add(message);
} catch(Exception e) {
return false;
}
}
T take () throws InterruptedException ;
T poll(long timeout, TimeUnit unit) throws InterruptedException;
void clear();
int size();
T peek();
/** LinkedBlockingDeque implementing MiniQueue */
static MiniQueue blocking() {
class MiniQueuedBlockingQueue extends LinkedBlockingDeque implements MiniQueue {};
return new MiniQueuedBlockingQueue<>();
}
/** Useful to reduce the starvation on weighted work stealing pools */
static PriorityMiniQueue priority() {
return new PriorityMiniQueue<>();
}
/** MiniQueue dropping all the messages */
static MiniQueue dropping() {
return new MiniQueue() {
@Override
public boolean add(T message) {
return false;
}
@Override
public T take() throws InterruptedException {
throw new UnsupportedOperationException("This queue drops all the message and so cannot provide the take() operation ");
}
@Override
public T poll(long timeout, TimeUnit unit) {
throw new UnsupportedOperationException("This queue drops all the message and so cannot provide the poll() operation ");
}
@Override
public void clear() {
}
@Override
public int size() {
return 0;
}
@Override
public T peek() {
return null;
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy