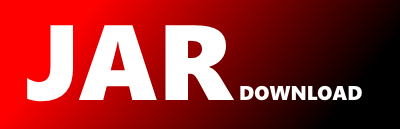
eu.lucaventuri.functional.Either3 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fibry Show documentation
Show all versions of fibry Show documentation
The first Java Actor System supporting fibers from Project Loom
package eu.lucaventuri.functional;
import eu.lucaventuri.common.ConsumerEx;
import eu.lucaventuri.common.Exceptions;
import java.util.Optional;
/** Functional Either, containing or one type or another */
public class Either3 {
private final L left;
private final R right;
private final O other;
private Either3(L left, R right, O other) {
this.left = left;
this.right = right;
this.other = other;
assert (left != null && right == null && other == null) ||
(left == null && right != null && other == null) ||
(left == null && right == null && other != null);
}
public static Either3 left(L value) {
Exceptions.assertAndThrow(value != null, "Left value is null!");
return new Either3(value, null, null);
}
public static Either3 right(R value) {
Exceptions.assertAndThrow(value != null, "Right value is null!");
return new Either3(null, value, null);
}
public static Either3 other(O value) {
Exceptions.assertAndThrow(value != null, "Other value is null!");
return new Either3(null, null, value);
}
public boolean isLeft() {
return left != null;
}
public boolean isRight() {
return right != null;
}
public boolean isOther() {
return other != null;
}
public L left() {
return left;
}
public R right() {
return right;
}
public O other() {
return other;
}
public Optional leftOpt() {
return Optional.ofNullable(left);
}
public Optional rightOpt() {
return Optional.ofNullable(right);
}
public Optional otherOpt() {
return Optional.ofNullable(other);
}
public void ifLeft(ConsumerEx consumer) throws E {
if (left != null)
consumer.accept(left);
}
public void ifRight(ConsumerEx consumer) throws E {
if (right != null)
consumer.accept(right);
}
public void ifOther(ConsumerEx consumer) throws E {
if (right != null)
consumer.accept(other);
}
public void ifEither(ConsumerEx consumerLeft, ConsumerEx consumerRight, ConsumerEx consumerOther) throws E {
if (left != null)
consumerLeft.accept(left);
else if (right!=null)
consumerRight.accept(right);
else
consumerOther.accept(other);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy