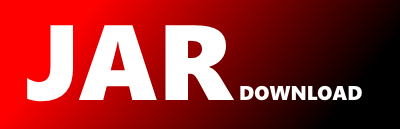
eu.mihosoft.vmf.vmftext.vmtemplates.unparser-formatter.vm Maven / Gradle / Ivy
The newest version!
#*
* Copyright 2017-2018 Michael Hoffer . All rights reserved.
* Copyright 2017-2018 Goethe Center for Scientific Computing, University Frankfurt. All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* If you use this software for scientific research then please cite the following publication(s):
*
* M. Hoffer, C. Poliwoda, & G. Wittum. (2013). Visual reflection library:
* a framework for declarative GUI programming on the Java platform.
* Computing and Visualization in Science, 2013, 16(4),
* 181–192. http://doi.org/10.1007/s00791-014-0230-y
*#
package ${modelPackageName}.unparser;
import java.io.PrintWriter;
import java.io.IOException;
import java.util.Objects;
import java.util.Optional;
import eu.mihosoft.vmf.runtime.core.VMF;
import ${modelPackageName}.ReadOnlyCodeElement;
import ${modelPackageName}.CodeElement;
import ${modelPackageName}.CodeRange;
public interface Formatter {
default void pushState() {}
default void acceptState() {}
default void rejectState() {}
default void pre( ${model.grammarName}ModelUnparser unparser, RuleInfo ruleInfo, PrintWriter w ){}
default void post(${model.grammarName}ModelUnparser unparser, RuleInfo ruleInfo, PrintWriter w ){}
static Formatter newDefaultFormatter() { return new DefaultFormatter();}
static Formatter newJavaStyleFormatter() { return new JavaStyleFormatter();}
static enum RuleType {
LEXER_RULE,
TERMINAL,
NONE
}
static final class RuleInfo {
private final CodeElement parentObject;
private final RuleType ruleType;
private final String ruleName;
private final String ruleText;
// consumed
private boolean consumed;
private RuleInfo(CodeElement parentObject, RuleType ruleType, String ruleName, String ruleText) {
this.parentObject = parentObject;
this.ruleType = ruleType;
this.ruleName = ruleName;
this.ruleText = ruleText;
}
public static RuleInfo emptyRule() {
return new RuleInfo(
new CodeElement() {
@Override
public CodeRange getCodeRange() {
throw new UnsupportedOperationException("Empty Code Element does not support this operation.");
}
@Override
public void setCodeRange(CodeRange codeRange) {
throw new UnsupportedOperationException("Empty Code Element does not support this operation.");
}
@Override
public ReadOnlyCodeElement asReadOnly() {
throw new UnsupportedOperationException("Empty Code Element does not support this operation.");
}
@Override
public VMF vmf() {
throw new UnsupportedOperationException("Empty Code Element does not support this operation.");
}
@Override
public CodeElement clone() {
throw new UnsupportedOperationException("Empty Code Element does not support this operation.");
}
}, RuleType.NONE, "","");
}
/**
* Calling this method consumes the current rule. This method only has an effect if called inside
* the {@code Formatter.pre()} method.
*/
public void consume() {
this.consumed = true;
}
boolean isConsumed() {
return this.consumed;
}
/*package private*/ static RuleInfo newRuleInfo(CodeElement parentObject, RuleType ruleType, String ruleName, String ruleText) {
return new RuleInfo(parentObject, ruleType, ruleName, ruleText);
}
public CodeElement getParentObject() {
return this.parentObject;
}
public RuleType getRuleType() {
return this.ruleType;
}
public Optional getRuleName() {
return Optional.ofNullable(this.ruleName);
}
public String getRuleText() {
return this.ruleText;
}
}
}
class DefaultFormatter implements Formatter {
public void post(${model.grammarName}ModelUnparser unparser, RuleInfo ruleInfo, PrintWriter w ) {
w.append(" ");
}
}
class JavaStyleFormatter implements Formatter {
private String last = "";
private String indent = "";
String getIndent() {
return indent;
}
void inc() {
indent+=" ";
}
void dec() {
if(indent.length() > 1) {
indent=indent.substring(2);
}
}
public void pre( ${model.grammarName}ModelUnparser unparser, RuleInfo ruleInfo, PrintWriter w ) {
if(Objects.equals(ruleInfo.getRuleText(), "}")) {
dec();
w.append('\n').append(getIndent());
}
}
public void post(${model.grammarName}ModelUnparser unparser, RuleInfo ruleInfo, PrintWriter w ) {
if(Objects.equals(ruleInfo.getRuleText(), "{")) {
inc();
w.append('\n').append(getIndent());
} else if(Objects.equals(ruleInfo.getRuleText(), ";")) {
w.append('\n').append(getIndent());
} else{
w.append(" ");
}
last = ruleInfo.getRuleText();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy