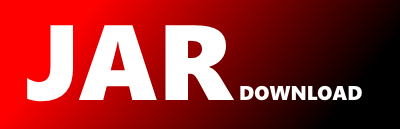
b4j.core.DefaultMetaInformation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of b4j Show documentation
Show all versions of b4j Show documentation
A Java library for accessing Bugzilla instances
/*
* This file is part of Bugzilla for Java.
*
* Bugzilla for Java is free software: you can redistribute it
* and/or modify it under the terms of version 3 of the GNU
* Lesser General Public License as published by the Free Software
* Foundation.
*
* Bugzilla for Java is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with Bugzilla for Java. If not, see
* .
*/
package b4j.core;
import java.io.File;
import java.io.FileReader;
import java.io.Reader;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
import org.apache.commons.configuration.Configuration;
import org.apache.commons.configuration.ConfigurationException;
import org.apache.commons.configuration.XMLConfiguration;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import rsbaselib.configuration.ConfigurationUtils;
import b4j.report.BugzillaReportGenerator;
/**
* Default implementation of meta information for a report generator.
* This class reads its data from a XML file.
* @author Ralph Schuster
*
*/
public class DefaultMetaInformation implements MetaInformation {
private static Logger log = LoggerFactory.getLogger(DefaultMetaInformation.class);
private List reports;
private SearchData searchData = new DefaultSearchData();
private Session bugzillaSession;
/**
* Default Constructor.
*/
public DefaultMetaInformation() {
}
/**
* Constructor with XML configuration file.
*/
public DefaultMetaInformation(File metaFile) throws Exception {
read(metaFile);
}
/**
* Reads the XML configuration file.
* @param metaFile - the XML file to read
* @throws Exception - if an error occurs
*/
public void read(File metaFile) throws Exception {
read(new FileReader(metaFile));
}
/**
* Reads and parses the XML configuration file from a stream reader.
* @param reader - a reader on the XML file.
* @throws ConfigurationException - if a configuration error occurs
*/
public void read(Reader reader) throws ConfigurationException {
XMLConfiguration config = new XMLConfiguration();
config.load(reader);
configure(config);
}
/**
* Configures the data from an XML configuration object.
* @param config - XML configuration
* @throws ConfigurationException - if a configuration error occurs
*/
@SuppressWarnings("unchecked")
public void configure(XMLConfiguration config) throws ConfigurationException {
// Create and configure bugzilla session
String className = null;
Configuration sessionCfg = config.configurationAt("bugzilla-session(0)");
bugzillaSession = (Session)ConfigurationUtils.load(sessionCfg, true);
// Reports to generate
reports = new ArrayList();
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy