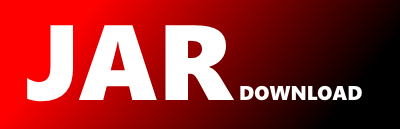
b4j.core.session.bugzilla.async.AsyncBugzillaUserRestClient Maven / Gradle / Ivy
/*
* This file is part of Bugzilla for Java.
*
* Bugzilla for Java is free software: you can redistribute it
* and/or modify it under the terms of version 3 of the GNU
* Lesser General Public License as published by the Free Software
* Foundation.
*
* Bugzilla for Java is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with Bugzilla for Java. If not, see
* .
*/
package b4j.core.session.bugzilla.async;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.concurrent.ExecutionException;
import org.codehaus.jettison.json.JSONObject;
import com.atlassian.jira.rest.client.RestClientException;
import com.atlassian.util.concurrent.Promise;
import b4j.core.User;
import b4j.core.session.bugzilla.BugzillaUserRestClient;
import b4j.core.session.bugzilla.json.BugzillaLoginParser;
import b4j.core.session.bugzilla.json.BugzillaUserParser;
/**
* The client responsible for getting classification information.
* @author ralph
* @since 2.0
*
*/
public class AsyncBugzillaUserRestClient extends AbstractAsyncRestClient implements BugzillaUserRestClient {
private BugzillaUserParser userParser;
private BugzillaLoginParser idParser;
/**
* Constructor.
*/
public AsyncBugzillaUserRestClient(AsyncBugzillaRestClient mainClient) {
super(mainClient, "User");
userParser = new BugzillaUserParser(getLazyRetriever());
idParser = new BugzillaLoginParser(getLazyRetriever());
}
/**
* {@inheritDoc}
*/
@Override
public User login(String user, String password) {
Map params = new HashMap();
params.put("login", user);
params.put("password", password);
params.put("remember", "False");
try {
LoginToken response = postAndParse("login", params, idParser).get();
getMainClient().setLoginToken(response);
return getUsers(response.getId()).get().iterator().next();
} catch (InterruptedException e) {
throw new RestClientException("Cannot login", e);
} catch (ExecutionException e) {
throw new RestClientException("Cannot login", e);
}
}
/**
* {@inheritDoc}
*/
@Override
public void logout() {
postAndParse("logout", (JSONObject)null, null);
getMainClient().setLoginToken(null);
}
/**
* {@inheritDoc}
*/
@Override
public Promise> getUsers(long... ids) {
List l = new ArrayList();
for (int i=0; i> getUsers(Collection ids) {
Map params = new HashMap();
params.put("ids", ids);
return postAndParse("get", params, userParser);
}
/**
* {@inheritDoc}
*/
@Override
public Promise> getUsersByName(String... names) {
List l = new ArrayList();
for (int i=0; i> getUsersByName(Collection names) {
Map params = new HashMap();
params.put("names", names);
return postAndParse("get", params, userParser);
}
/**
* Required for parsing the login response.
* @author ralph
* @since 2.0.3
*/
public static class LoginToken {
private Long id;
private String token;
/**
* Constructor.
* @param id
* @param token
*/
public LoginToken(Long id, String token) {
setId(id);
setToken(token);
}
/**
* Returns the id.
* @return the id
*/
public Long getId() {
return id;
}
/**
* Sets the id.
* @param id the id to set
*/
public void setId(Long id) {
this.id = id;
}
/**
* Returns the token.
* @return the token
*/
public String getToken() {
return token;
}
/**
* Sets the token.
* @param token the token to set
*/
public void setToken(String token) {
this.token = token;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy