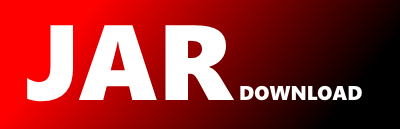
csv.util.ObjectReader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of csv Show documentation
Show all versions of csv Show documentation
A library for easily accessing CSV, Excel and and other table-like data from Java
/*
* This file is part of CSV/Excel Utility Package.
*
* CSV/Excel Utility Package is free software: you can redistribute it
* and/or modify it under the terms of version 3 of the GNU
* Lesser General Public License as published by the Free Software
* Foundation.
*
* CSV/Excel Utility Package is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with CSV/Excel Utility Package. If not, see
* .
*/
package csv.util;
import java.util.Iterator;
import csv.TableReader;
/**
* Reads objects from a table.
* This class is different to {@link BeanReader} as it asks a converter to convert the row.
*
* // Create an instance of your table reader
* TableReader tableReader = ...;
*
* // Get an instance of your row converter
* RowConverter<MyClass> converter = new MyClassConverter();
*
* // Now read from the table stream
* ObjectReader<MyClass> reader = new ObjectReader(tableReader, converter, true);
* Object tableHeader[] = reader.getTableHeader();
* while (reader.hasNext()) {
* MyClass myObject = reader.next();
*
* // do something...
* }
*
* // Close the reader
* reader.close();
*
* @author ralph
*
*/
public class ObjectReader implements Iterator, Iterable {
protected TableReader reader;
protected Iterator
© 2015 - 2024 Weber Informatics LLC | Privacy Policy