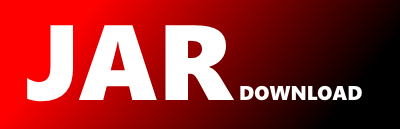
rs.data.file.storage.XmlStorageStrategy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of data-file Show documentation
Show all versions of data-file Show documentation
Library for file-based data access
The newest version!
/*
* This file is part of RS Library (Data File Library).
*
* RS Library is free software: you can redistribute it
* and/or modify it under the terms of version 3 of the GNU
* Lesser General Public License as published by the Free Software
* Foundation.
*
* RS Library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with RS Library. If not, see
* .
*/
package rs.data.file.storage;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStreamWriter;
import java.io.Serializable;
import java.io.Writer;
import java.lang.reflect.InvocationTargetException;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
import org.apache.commons.beanutils.PropertyUtils;
import org.apache.commons.configuration2.HierarchicalConfiguration;
import org.apache.commons.configuration2.XMLConfiguration;
import org.apache.commons.io.Charsets;
import rs.baselib.bean.IBean;
import rs.baselib.configuration.ConfigurationUtils;
import rs.baselib.lang.LangUtils;
import rs.data.api.IDaoFactory;
import rs.data.api.bo.IGeneralBO;
import rs.data.api.dao.IGeneralDAO;
/**
* Storage strategy for XML files.
* @param type of ID for Business Objects to be managed
* @param type of Business Object the strategy manages
* @author ralph
*
*/
public class XmlStorageStrategy> extends AbstractStorageStrategy {
/**
* Constructor.
* @param daoFactory - the DAO factory this strategy belongs to
*/
public XmlStorageStrategy(IDaoFactory daoFactory) {
super(daoFactory);
}
/**
* {@inheritDoc}
*/
@Override
public void load(T bo, K id, File specifier) throws IOException {
// Find all relevant property names
Collection propertyNames = new ArrayList(getPropertyNames(bo));
propertyNames.add("id");
try {
XMLConfiguration cfg = ConfigurationUtils.getXmlConfiguration(specifier);
// Removed in 2.0: cfg.setListDelimiter((char)0);
for (String name : propertyNames) {
try {
HierarchicalConfiguration> subConfig = cfg.configurationAt(name+"(0)");
bo.set(name, loadValue(subConfig));
} catch (IllegalArgumentException e) {
bo.set(name, null);
}
}
} catch (Exception e) {
throw new IOException("Cannot load XML file", e);
}
}
/**
* Internal helper to load a sub configuration.
* Descendants shall not override this but several specific helper methods.
* @param cfg the tag to be loaded
* @return th eobject represented by this tag
* @see #loadBusinessObject(Class, HierarchicalConfiguration)
* @see #loadCollection(Class, HierarchicalConfiguration)
* @see #loadMap(Class, HierarchicalConfiguration)
* @see #loadBean(Class, HierarchicalConfiguration)
* @see #loadSerialized(Class, HierarchicalConfiguration)
* @throws IOException - the I/O exception when operating on a filesystem
* @throws ClassNotFoundException - when class could not be found
* @throws InstantiationException - when object cannot be instantiated
* @throws IllegalAccessException - when constructor is not accessible
* @throws InvocationTargetException - when constructor threw an exception
* @throws NoSuchMethodException - when constructor does not exist
*/
protected Object loadValue(HierarchicalConfiguration> cfg) throws IOException, ClassNotFoundException, InstantiationException, IllegalAccessException, InvocationTargetException, NoSuchMethodException {
String className = cfg.getString("[@class]");
if ((className != null) && !className.isEmpty()) {
Class> clazz = LangUtils.forName(className);
Object rc = null;
if (IGeneralBO.class.isAssignableFrom(clazz)) {
rc = loadBusinessObject(clazz, cfg);
} else if (Collection.class.isAssignableFrom(clazz)) {
rc = loadCollection(clazz, cfg);
} else if (Map.class.isAssignableFrom(clazz)) {
rc = loadMap(clazz, cfg);
} else if (IBean.class.isAssignableFrom(clazz)) {
rc = loadBean(clazz, cfg);
} else {
rc = loadSerialized(clazz, cfg);
if (rc == null) {
throw new RuntimeException("Cannot unserialize property: "+className+": "+cfg.getString(""));
}
}
return rc;
} else {
return null;
}
}
/**
* Loads a {@link IGeneralBO business object} from the XML tag.
* @param clazz class to be loaded
* @param cfg configuration of class
* @return the loaded object
* @throws IOException - the I/O exception when operating on a filesystem
* @throws ClassNotFoundException - when class could not be found
* @throws InstantiationException - when object cannot be instantiated
* @throws IllegalAccessException - when constructor is not accessible
* @throws InvocationTargetException - when constructor threw an exception
* @throws NoSuchMethodException - when constructor does not exist
*/
@SuppressWarnings("unchecked")
protected IGeneralBO> loadBusinessObject(Class> clazz, HierarchicalConfiguration> cfg) throws IOException, ClassNotFoundException, InstantiationException, IllegalAccessException, InvocationTargetException, NoSuchMethodException {
Serializable id = (Serializable)loadValue(cfg.configurationAt("refid(0)"));
IDaoFactory factory = getDaoFactory();
if (factory != null) {
IGeneralDAO, ? extends IGeneralBO extends Serializable>> dao = factory.getDaoFor((Class extends IGeneralBO extends Serializable>>)clazz);
if (dao != null) return dao.findById(id);
}
return null;
}
/**
* Loads a collection from the XML tag.
* @param clazz class to be loaded
* @param cfg configuration of class
* @return the loaded collection
* @throws IOException - the I/O exception when operating on a filesystem
* @throws ClassNotFoundException - when class could not be found
* @throws InstantiationException - when object cannot be instantiated
* @throws IllegalAccessException - when constructor is not accessible
* @throws InvocationTargetException - when constructor threw an exception
* @throws NoSuchMethodException - when constructor does not exist
*/
@SuppressWarnings("unchecked")
protected Collection> loadCollection(Class> clazz, HierarchicalConfiguration> cfg) throws IOException, ClassNotFoundException, InstantiationException, IllegalAccessException, InvocationTargetException, NoSuchMethodException {
Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy