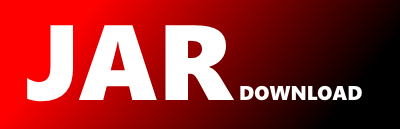
rs.data.file.util.DefaultFilenameStrategy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of data-file Show documentation
Show all versions of data-file Show documentation
Library for file-based data access
The newest version!
/*
* This file is part of RS Library (Data File Library).
*
* RS Library is free software: you can redistribute it
* and/or modify it under the terms of version 3 of the GNU
* Lesser General Public License as published by the Free Software
* Foundation.
*
* RS Library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with RS Library. If not, see
* .
*/
package rs.data.file.util;
import java.io.File;
import java.io.IOException;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
/**
* Default implementation of a filename strategy.
* @author ralph
*
*/
public class DefaultFilenameStrategy implements IFilenameStrategy {
/** The parent directory of a file */
private File parentDir;
/** The prefix of a filename */
private String prefix;
/** The suffix of a filename */
private String suffix;
/**
* Constructor.
* Data will be stored in current working directory, sub-directory data with suffix ".data".
*/
public DefaultFilenameStrategy() {
this(new File(System.getProperty("user.dir"), DEFAULT_DATA_DIR), null, DEFAULT_DATA_SUFFIX);
}
/**
* Constructor.
* @param parentDir - the directory to store at
* @param prefix - the file prefix to be used
* @param suffix - the suffix to be used
*/
public DefaultFilenameStrategy(String parentDir, String prefix, String suffix) {
this(new File(parentDir), prefix, suffix);
}
/**
* Constructor.
* @param parentDir - the directory to store at
* @param prefix - the file prefix to be used
* @param suffix - the suffix to be used
*/
public DefaultFilenameStrategy(File parentDir, String prefix, String suffix) {
setParentDir(parentDir);
setPrefix(prefix);
setSuffix(suffix);
}
/**
* Returns the {@link #parentDir}.
* @return the parentDir
*/
public File getParentDir() {
return parentDir;
}
/**
* Sets the {@link #parentDir}.
* @param parentDir the parentDir to set
*/
public void setParentDir(File parentDir) {
this.parentDir = parentDir;
}
/**
* Returns the {@link #prefix}.
* @return the prefix
*/
public String getPrefix() {
return prefix;
}
/**
* Sets the {@link #prefix}.
* @param prefix the prefix to set
*/
public void setPrefix(String prefix) {
this.prefix = prefix;
}
/**
* Returns the {@link #suffix}.
* @return the suffix
*/
public String getSuffix() {
return suffix;
}
/**
* Sets the {@link #suffix}.
* @param suffix the suffix to set
*/
public void setSuffix(String suffix) {
this.suffix = suffix;
}
/**
* {@inheritDoc}
*/
@Override
public File getFile(K key) throws IOException {
File parentDir = getParentDir();
if (!parentDir.exists()) {
if (!createParent(parentDir)) {
throw new IOException("Cannot create parent directory: "+parentDir.getAbsolutePath());
}
}
String filename = "";
String prefix = getPrefix();
if (prefix != null) filename += prefix;
filename += key.toString();
String suffix = getSuffix();
if (suffix != null) filename += suffix;
return new File(parentDir, filename);
}
/**
* Called when a new file is required to be created.
* Creates the parent dir.
* @param dir the directory to be created
* @return true
when the parent was created or exists
*/
protected boolean createParent(File dir) {
if (!dir.exists()) return dir.mkdirs();
return true;
}
/**
* Returns whether the given file matches the strategy.
* @param file file to be checked
* @return true
when strategy matches
*/
public boolean matches(File file) {
if (!file.getParentFile().equals(getParentDir())) return false;
return matchesFilename(file.getName());
}
/**
* Returns true when the given filename (last path component!) matches this strategy.
* @param filename filename to be matched
* @return true
when strategy matches
*/
public boolean matchesFilename(String filename) {
String prefix = getPrefix();
if (prefix != null) {
if (!filename.startsWith(prefix)) return false;
}
String suffix = getSuffix();
if (suffix != null) {
if (!filename.endsWith(suffix)) return false;
}
return true;
}
/**
* {@inheritDoc}
*/
@Override
public Collection getFiles() {
List rc = new ArrayList();
if (getParentDir().exists() && getParentDir().isDirectory()) {
for (File child : getParentDir().listFiles()) {
if (matchesFilename(child.getName()) && child.canRead()) rc.add(child);
}
Collections.sort(rc);
}
return rc;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy