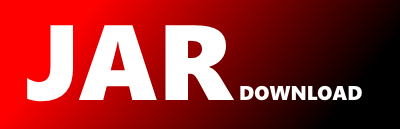
rs.data.hibernate.bo.AbstractHibernateBO Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of data-hibernate Show documentation
Show all versions of data-hibernate Show documentation
Hibernate extension for Data Access classes
/**
*
*/
package rs.data.hibernate.bo;
import java.io.Serializable;
import org.hibernate.Session;
import org.hibernate.proxy.HibernateProxy;
import org.hibernate.proxy.LazyInitializer;
import rs.data.hibernate.HibernateDaoMaster;
import rs.data.impl.bo.AbstractBO;
import rs.data.impl.dto.GeneralDTO;
/**
* Abstract implementation for hibernate BO.
* @author ralph
*
*/
public abstract class AbstractHibernateBO> extends AbstractBO {
/**
* Serial UID.
*/
private static final long serialVersionUID = -248221407299395538L;
/**
* Constructor.
*/
public AbstractHibernateBO() {
this(null);
}
/**
* Constructor.
*/
public AbstractHibernateBO(T transferObject) {
super(transferObject);
}
/**
* Returns the DAO master.
* @return the DAO master
*/
protected HibernateDaoMaster getDaoMaster() {
return (HibernateDaoMaster)getDao().getDaoMaster();
}
/**
* Returns a Hibernate session.
* @return the session
*/
protected Session getSession() {
return getDaoMaster().getSession();
}
/**
* Returns the transferObject.
* @return the transferObject
*/
public T getTransferObject() {
boolean needsInit = false;
T t = super.getTransferObject();
if (t instanceof HibernateProxy) {
LazyInitializer initializer = ((HibernateProxy)t).getHibernateLazyInitializer();
if (initializer != null) needsInit = initializer.isUninitialized();
}
if (needsInit) t = initialize();
return t;
}
/**
* Returns the session-attached transferObject.
* @return the session attached transferObject
*/
@SuppressWarnings("unchecked")
public T getAttachedTransferObject() {
// First check whether T is still attached
T t = super.getTransferObject();
if (t instanceof HibernateProxy) {
LazyInitializer initializer = ((HibernateProxy)t).getHibernateLazyInitializer();
if (initializer != null) {
if (!initializer.getSession().isOpen()) {
t = (T)getSession().get(getTransferClass(), getId());
setTransferObject(t);
}
}
return t;
}
t = (T)getSession().get(getTransferClass(), getId());
setTransferObject(t);
return t;
}
/**
* {@inheritDoc}
*/
@Override
public K getId() {
// Do not initialize transfer object
return super.getTransferObject().getId();
}
/**
* Initializes the DTO.
*/
@SuppressWarnings("unchecked")
protected T initialize() {
T t = super.getTransferObject();
try {
beginTx();
if (t instanceof HibernateProxy) {
LazyInitializer initializer = ((HibernateProxy)t).getHibernateLazyInitializer();
if (initializer != null) {
if (initializer.getSession() == null || !initializer.getSession().isOpen()) {
t = (T)getSession().get(getTransferClass(), getId());
super.setTransferObject(t);
}
}
}
commitTx();
} catch (Exception e) {
getLog().error("init problem:", e);
try {
rollbackTx();
} catch (Exception e2) {
throw new RuntimeException("Cannot rollback exception", e2);
}
throw new RuntimeException("Cannot initialize DTO", e);
}
return t;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy