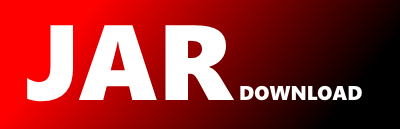
com.sportdataapi.client.PlayersClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sportdata-api-client Show documentation
Show all versions of sportdata-api-client Show documentation
A simple sportdataapi.com client.
The newest version!
/**
*
*/
package com.sportdataapi.client;
import java.util.List;
import javax.ws.rs.NotFoundException;
import javax.ws.rs.client.WebTarget;
import javax.ws.rs.core.GenericType;
import com.sportdataapi.data.Player;
import com.sportdataapi.util.AbstractClient;
import com.sportdataapi.util.Response;
/**
* The players client.
* Attention! You shall never create this client directly but use {@link SoccerClient#players()} instead.
* @author ralph
*
*/
public class PlayersClient extends AbstractClient {
/**
* Constructor.
* @param target - the target to request
*/
public PlayersClient(WebTarget target) {
super(target.path("players"));
}
/**
* Request and return the list of players.
* @param countryId - ID of country of players
* @return list of players
*/
public List list(int countryId) {
return list(countryId, 0, 0);
}
/**
* Request and return the list of players.
* @param countryId - ID of country of players
* @param minAge - minimum age of players
* @return list of players
*/
public List listMinAge(int countryId, int minAge) {
return list(countryId, minAge, 0);
}
/**
* Request and return the list of players.
* @param countryId - ID of country of players
* @param maxAge - maximum age of players
* @return list of players
*/
public List listMaxAge(int countryId, int maxAge) {
return list(countryId, 0, maxAge);
}
/**
* Request and return the list of players.
* @param countryId - ID of country of players
* @param minAge - minimum age of players
* @param maxAge - maximum age of players
* @return list of players
*/
public List list(int countryId, int minAge, int maxAge) {
WebTarget target = getTarget().queryParam("country_id", ""+countryId);
if (minAge > 0) target = target.queryParam("min_age", ""+minAge);
if (maxAge > 0) target = target.queryParam("max_age", ""+maxAge);
Response> response = registerRequest(target).request().get(new GenericType>>() {});
return response.getData();
}
/**
* Returns the player with given id.
* @param id - ID of player
* @return the player or {@code null}
*/
public Player get(int id) {
try {
Response response = registerRequest(getTarget().path(""+id)).request().get(new GenericType>() {});
return response.getData();
} catch (NotFoundException e) {
return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy