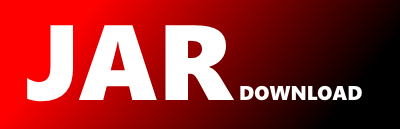
eu.stamp.project.assertfixer.util.Util Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of assert-fixer Show documentation
Show all versions of assert-fixer Show documentation
This tool aims at repairing the assertion inside JUnit tests.
package eu.stamp.project.assertfixer.util;
import spoon.reflect.code.CtInvocation;
import spoon.reflect.declaration.CtType;
import java.util.ArrayList;
import java.util.List;
import java.util.function.Function;
import java.util.function.Predicate;
/**
* Created by Benjamin DANGLOT
* [email protected]
* on 24/05/17
*/
public class Util {
public static final List assertionErrors = new ArrayList<>();
static {
assertionErrors.add("java.lang.AssertionError");
assertionErrors.add("org.junit.internal.ArrayComparisonFailure");
}
public static final Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy