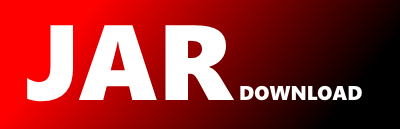
org.evosuite.utils.Randomness Maven / Gradle / Ivy
The newest version!
/**
* Copyright (C) 2010-2018 Gordon Fraser, Andrea Arcuri and EvoSuite
* contributors
*
* This file is part of EvoSuite.
*
* EvoSuite is free software: you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, either version 3.0 of the License, or
* (at your option) any later version.
*
* EvoSuite is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with EvoSuite. If not, see .
*/
package org.evosuite.utils;
import java.io.Serializable;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Random;
import org.evosuite.Properties;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* Unique random number accessor
*
* @author Gordon Fraser
*/
public class Randomness implements Serializable {
private static final long serialVersionUID = -5934455398558935937L;
private static final Logger logger = LoggerFactory.getLogger(Randomness.class);
private static long seed = 0;
private static Random random = null;
private static Randomness instance = new Randomness();
private Randomness() {
Long seed_parameter = Properties.RANDOM_SEED;
if (seed_parameter != null) {
seed = seed_parameter;
logger.info("Random seed: {}", seed);
} else {
seed = System.currentTimeMillis();
logger.info("No seed given. Using {}.", seed);
}
random = new MersenneTwister(seed);
}
/**
*
* Getter for the field instance
.
*
*
* @return a {@link org.evosuite.utils.Randomness} object.
*/
public static Randomness getInstance() {
if (instance == null) {
instance = new Randomness();
}
return instance;
}
/**
*
* nextBoolean
*
*
* @return a boolean.
*/
public static boolean nextBoolean() {
return random.nextBoolean();
}
/**
*
* nextInt
*
*
* @param max
* a int.
* @return a int.
*/
public static int nextInt(int max) {
return random.nextInt(max);
}
public static double nextGaussian() {
return random.nextGaussian();
}
/**
*
* nextInt
*
*
* @param min
* a int.
* @param max
* a int.
* @return a int.
*/
public static int nextInt(int min, int max) {
return random.nextInt(max - min) + min;
}
/**
*
* nextInt
*
*
* @return a int.
*/
public static int nextInt() {
return random.nextInt();
}
/**
*
* nextChar
*
*
* @return a char.
*/
public static char nextChar() {
return (char) (nextInt(32, 128));
//return random.nextChar();
}
/**
*
* nextShort
*
*
* @return a short.
*/
public static short nextShort() {
return (short) (random.nextInt(2 * 32767) - 32767);
}
/**
*
* nextLong
*
*
* @return a long.
*/
public static long nextLong() {
return random.nextLong();
}
/**
*
* nextByte
*
*
* @return a byte.
*/
public static byte nextByte() {
return (byte) (random.nextInt(256) - 128);
}
/**
*
* returns a randomly generated double in the range [0,1]
*
*
* @return a double between 0.0 and 1.0
*/
public static double nextDouble() {
return random.nextDouble();
}
/**
*
* nextDouble
*
*
* @param min
* a double.
* @param max
* a double.
* @return a double.
*/
public static double nextDouble(double min, double max) {
return min + (random.nextDouble() * (max - min));
}
/**
*
* nextFloat
*
*
* @return a float.
*/
public static float nextFloat() {
return random.nextFloat();
}
/**
*
* Setter for the field seed
.
*
*
* @param seed
* a long.
*/
public static void setSeed(long seed) {
Randomness.seed = seed;
random.setSeed(seed);
}
/**
*
* Getter for the field seed
.
*
*
* @return a long.
*/
public static long getSeed() {
return seed;
}
/**
*
* choice
*
*
* @param list
* a {@link java.util.List} object.
* @param
* a T object.
* @return a T object or null
if list
is empty.
*/
public static T choice(List list) {
if (list.isEmpty())
return null;
int position = random.nextInt(list.size());
return list.get(position);
}
/**
*
* choice
*
*
* @param set
* a {@link java.util.Collection} object.
* @param
* a T object.
* @return a T object or null
if set
is empty.
*/
@SuppressWarnings("unchecked")
public static T choice(Collection set) {
if (set.isEmpty())
return null;
int position = random.nextInt(set.size());
return (T) set.toArray()[position];
}
/**
*
* choice
*
*
* @param elements
* a T object.
* @param
* a T object.
* @return a T object or null
if elements.length
is zero.
*/
public static T choice(T... elements) {
if (elements.length == 0)
return null;
int position = random.nextInt(elements.length);
return elements[position];
}
/**
*
* shuffle
*
*
* @param list
* a {@link java.util.List} object.
*/
public static void shuffle(List> list) {
Collections.shuffle(list, random);
}
/**
*
* nextString
*
*
* @param length
* a int.
* @return a {@link java.lang.String} object.
*/
public static String nextString(int length) {
char[] characters = new char[length];
for (int i = 0; i < length; i++)
characters[i] = nextChar();
return new String(characters);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy