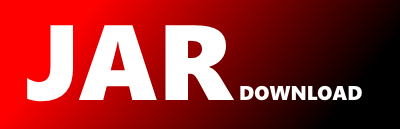
eu.tneitzel.argparse4j.impl.Arguments Maven / Gradle / Ivy
Show all versions of argparse4j Show documentation
/*
* Copyright (C) 2011 Tatsuhiro Tsujikawa
*
* Permission is hereby granted, free of charge, to any person
* obtaining a copy of this software and associated documentation
* files (the "Software"), to deal in the Software without
* restriction, including without limitation the rights to use, copy,
* modify, merge, publish, distribute, sublicense, and/or sell copies
* of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be
* included in all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS
* BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN
* ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN
* CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
package eu.tneitzel.argparse4j.impl;
import java.util.List;
import eu.tneitzel.argparse4j.impl.action.AppendArgumentAction;
import eu.tneitzel.argparse4j.impl.action.AppendConstArgumentAction;
import eu.tneitzel.argparse4j.impl.action.CountArgumentAction;
import eu.tneitzel.argparse4j.impl.action.HelpArgumentAction;
import eu.tneitzel.argparse4j.impl.action.StoreArgumentAction;
import eu.tneitzel.argparse4j.impl.action.StoreConstArgumentAction;
import eu.tneitzel.argparse4j.impl.action.StoreFalseArgumentAction;
import eu.tneitzel.argparse4j.impl.action.StoreTrueArgumentAction;
import eu.tneitzel.argparse4j.impl.action.VersionArgumentAction;
import eu.tneitzel.argparse4j.impl.choice.RangeArgumentChoice;
import eu.tneitzel.argparse4j.impl.type.BooleanArgumentType;
import eu.tneitzel.argparse4j.impl.type.CaseInsensitiveEnumNameArgumentType;
import eu.tneitzel.argparse4j.impl.type.CaseInsensitiveEnumStringArgumentType;
import eu.tneitzel.argparse4j.impl.type.EnumArgumentType;
import eu.tneitzel.argparse4j.impl.type.EnumStringArgumentType;
import eu.tneitzel.argparse4j.impl.type.FileArgumentType;
import eu.tneitzel.argparse4j.impl.type.ReflectArgumentType;
import eu.tneitzel.argparse4j.inf.Argument;
import eu.tneitzel.argparse4j.inf.ArgumentParser;
import eu.tneitzel.argparse4j.inf.FeatureControl;
/**
*
* This class provides useful shortcuts and constants.
*
*
*
* They are mainly used to specify parameter to {@link Argument} object.
*
*/
public final class Arguments {
/**
* Intentionally made private to avoid instantiation in application code.
*/
private Arguments() {
}
/**
*
* Creates new range constrained choice.
*
*
* The value specified in command line will be checked to see whether it
* fits in given range [min, max], inclusive.
*
*
* @param
* The type of the values in the range
* @param min
* The lower bound of the range, inclusive.
* @param max
* The upper bound of the range, inclusive.
* @return {@link RangeArgumentChoice} object.
*/
public static > RangeArgumentChoice range(T min,
T max) {
return new RangeArgumentChoice<>(min, max);
}
private static final StoreArgumentAction store_ = new StoreArgumentAction();
private static final StoreTrueArgumentAction storeTrue_ = new StoreTrueArgumentAction();
private static final StoreFalseArgumentAction storeFalse_ = new StoreFalseArgumentAction();
private static final StoreConstArgumentAction storeConst_ = new StoreConstArgumentAction();
private static final AppendArgumentAction append_ = new AppendArgumentAction();
private static final AppendConstArgumentAction appendConst_ = new AppendConstArgumentAction();
private static final HelpArgumentAction help_ = new HelpArgumentAction();
private static final VersionArgumentAction version_ = new VersionArgumentAction();
private static final CountArgumentAction count_ = new CountArgumentAction();
/**
*
* The value of {@link FeatureControl#SUPPRESS}.
*
*
*
* If value is used with {@link Argument#setDefault(FeatureControl)}, no
* attribute is added if the command line argument was not present.
* Otherwise, the default value, which defaults to null, will be added to
* the object, regardless of the presence of command line argument, returned
* by {@link ArgumentParser#parseArgs(String[])}.
*
*/
public static final FeatureControl SUPPRESS = FeatureControl.SUPPRESS;
/**
* Returns store action.
*
* @return {@link StoreArgumentAction} object.
*/
public static StoreArgumentAction store() {
return store_;
}
/**
*
* Returns storeTrue action.
*
*
*
* If this action is used, the value specified using
* {@link Argument#nargs(int)} will be ignored.
*
*
* @return {@link StoreTrueArgumentAction} object.
*/
public static StoreTrueArgumentAction storeTrue() {
return storeTrue_;
}
/**
*
* Returns storeFalse action.
*
*
*
* If this action is used, the value specified using
* {@link Argument#nargs(int)} will be ignored.
*
*
* @return {@link StoreFalseArgumentAction} object.
*/
public static StoreFalseArgumentAction storeFalse() {
return storeFalse_;
}
/**
*
* Returns storeConst action.
*
*
*
* If this action is used, the value specified using
* {@link Argument#nargs(int)} will be ignored.
*
*
* @return {@link StoreConstArgumentAction} object.
*/
public static StoreConstArgumentAction storeConst() {
return storeConst_;
}
/**
*
* Returns append action.
*
*
* If this action is used, the attribute will be of type {@link List}. If
* used with {@link Argument#nargs(int)}, the element of List will be List.
* This is because {@link Argument#nargs(int)} produces List.
*
*
* @return {@link AppendArgumentAction} object.
*/
public static AppendArgumentAction append() {
return append_;
}
/**
*
* Returns appendConst action.
*
*
* If this action is used, the value specified using
* {@link Argument#nargs(int)} will be ignored.
*
*
* @return {@link AppendConstArgumentAction} object.
*/
public static AppendConstArgumentAction appendConst() {
return appendConst_;
}
/**
*
* Returns help action.
*
*
* This is used for an option printing help message. Please note that this
* action terminates program after printing help message.
*
*
* @return {@link HelpArgumentAction} object.
*/
public static HelpArgumentAction help() {
return help_;
}
/**
*
* Returns version action.
*
*
* This is used for an option printing version message. Please note that
* this action terminates program after printing version message.
*
*
* @return {@link VersionArgumentAction} object.
*/
public static VersionArgumentAction version() {
return version_;
}
/**
*
* Returns count action.
*
*
* This action counts the number of occurrence of the option. This action
* does not consume argument.
*
*
* @return {@link CountArgumentAction} object.
*/
public static CountArgumentAction count() {
return count_;
}
/**
*
* Returns {@link EnumArgumentType} with given enum {@code type}.
*
*
* Since enum does not have a constructor with string argument, you cannot
* use {@link Argument#type(Class)}. Instead use this convenient function.
*
*
* @deprecated
*
* @param
* The type of the enum
* @param type
* The enum type
* @return {@link EnumArgumentType} object
*/
@Deprecated
public static > EnumArgumentType enumType(Class type) {
return new EnumArgumentType<>(type);
}
/**
*
* Returns new {@link FileArgumentType} object.
*
*
* @return {@link FileArgumentType} object
*/
public static FileArgumentType fileType() {
return new FileArgumentType();
}
/**
*
* Returns {@link EnumStringArgumentType} with given enum {@code type}.
*
*
* Uses {@link Enum#toString()} instead of {@link Enum#name()} as the String
* representation of the enum. For enums that do not override
* {@link Enum#toString()}, this behaves the same as
* {@link ReflectArgumentType} or just use {@link Argument#type(Class)}.
*
*
* @param
* The type of the enum
* @param type
* The enum type
* @return {@link EnumStringArgumentType} object
*/
public static > EnumStringArgumentType enumStringType(
Class type) {
return new EnumStringArgumentType<>(type);
}
/**
*
* Returns {@link CaseInsensitiveEnumNameArgumentType} with given enum
* {@code type}.
*
*
* Uses {@link Enum#name()} as the String representation of the enum.
*
*
* @param
* The type of the enum
* @param type
* The enum type
* @return {@link CaseInsensitiveEnumNameArgumentType} object
* @since 0.8.0
*/
public static > CaseInsensitiveEnumNameArgumentType
caseInsensitiveEnumType(Class type) {
return new CaseInsensitiveEnumNameArgumentType<>(type);
}
/**
*
* Returns {@link CaseInsensitiveEnumStringArgumentType} with given enum
* {@code type}.
*
*
* Uses {@link Enum#toString()} instead of {@link Enum#name()} as the String
* representation of the enum. For enums that do not override
* {@link Enum#toString()}, this behaves the same as
* {@link CaseInsensitiveEnumNameArgumentType}.
*
*
* @param
* The type of the enum
* @param type
* The enum type
* @return {@link CaseInsensitiveEnumStringArgumentType} object
* @since 0.8.0
*/
public static > CaseInsensitiveEnumStringArgumentType
caseInsensitiveEnumStringType(Class type) {
return new CaseInsensitiveEnumStringArgumentType<>(type);
}
/**
*
* Returns {@link BooleanArgumentType} with "true" as true value, and
* "false" as false value.
*
*
* Read {@link BooleanArgumentType} documentation to know the difference
* between the use of {@link BooleanArgumentType} and passing
* {@link Boolean} class to {@link Argument#type(Class)}.
*
*
* @return The BooleanArgumentType object
* @since 0.7.0
*/
public static BooleanArgumentType booleanType() {
return new BooleanArgumentType();
}
/**
*
* Returns {@link BooleanArgumentType} with given true/false values.
*
*
* Read {@link BooleanArgumentType} documentation to know the difference
* between the use of {@link BooleanArgumentType} and passing
* {@link Boolean} class to {@link Argument#type(Class)}.
*
*
* @param trueValue
* string used as true value
* @param falseValue
* string used as false value
* @return The BooleanArgumentType object
* @since 0.7.0
*/
public static BooleanArgumentType booleanType(String trueValue,
String falseValue) {
return new BooleanArgumentType(trueValue, falseValue);
}
}