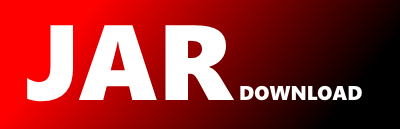
eu.toolchain.serializer.perftests.AutoMatterSerializedObjectBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tiny-serializer-perftests Show documentation
Show all versions of tiny-serializer-perftests Show documentation
Performance tests for TinySerializer.
The newest version!
package eu.toolchain.serializer.perftests;
import io.norberg.automatter.AutoMatter;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import javax.annotation.Generated;
@Generated("io.norberg.automatter.processor.AutoMatterProcessor")
public final class AutoMatterSerializedObjectBuilder {
private int version;
private String field;
private Map map;
private List list;
private Map> optionalMap;
private Set set;
private int[][][] deeplyNested;
public AutoMatterSerializedObjectBuilder() {
}
private AutoMatterSerializedObjectBuilder(AutoMatterSerializedObject v) {
this.version = v.version();
this.field = v.field();
Map _map = v.map();
this.map = (_map == null) ? null : new HashMap(_map);
List _list = v.list();
this.list = (_list == null) ? null : new ArrayList(_list);
Map> _optionalMap = v.optionalMap();
this.optionalMap = (_optionalMap == null) ? null : new HashMap>(_optionalMap);
Set _set = v.set();
this.set = (_set == null) ? null : new HashSet(_set);
this.deeplyNested = v.deeplyNested();
}
private AutoMatterSerializedObjectBuilder(AutoMatterSerializedObjectBuilder v) {
this.version = v.version;
this.field = v.field;
this.map = (v.map == null) ? null : new HashMap(v.map);
this.list = (v.list == null) ? null : new ArrayList(v.list);
this.optionalMap = (v.optionalMap == null) ? null : new HashMap>(v.optionalMap);
this.set = (v.set == null) ? null : new HashSet(v.set);
this.deeplyNested = v.deeplyNested;
}
public int version() {
return version;
}
public AutoMatterSerializedObjectBuilder version(int version) {
this.version = version;
return this;
}
public String field() {
return field;
}
public AutoMatterSerializedObjectBuilder field(String field) {
if (field == null) {
throw new NullPointerException("field");
}
this.field = field;
return this;
}
public Map map() {
if (this.map == null) {
this.map = new HashMap();
}
return map;
}
public AutoMatterSerializedObjectBuilder map(Map extends String, ? extends String> map) {
if (map == null) {
throw new NullPointerException("map");
}
for (Map.Entry extends String, ? extends String> entry : map.entrySet()) {
if (entry.getKey() == null) {
throw new NullPointerException("map: null key");
}
if (entry.getValue() == null) {
throw new NullPointerException("map: null value");
}
}
this.map = new HashMap(map);
return this;
}
public AutoMatterSerializedObjectBuilder map(String k1, String v1) {
if (k1 == null) {
throw new NullPointerException("map: k1");
}
if (v1 == null) {
throw new NullPointerException("map: v1");
}
map = new HashMap();
map.put(k1, v1);
return this;
}
public AutoMatterSerializedObjectBuilder map(String k1, String v1, String k2, String v2) {
map(k1, v1);
if (k2 == null) {
throw new NullPointerException("map: k2");
}
if (v2 == null) {
throw new NullPointerException("map: v2");
}
map.put(k2, v2);
return this;
}
public AutoMatterSerializedObjectBuilder map(String k1, String v1, String k2, String v2, String k3, String v3) {
map(k1, v1, k2, v2);
if (k3 == null) {
throw new NullPointerException("map: k3");
}
if (v3 == null) {
throw new NullPointerException("map: v3");
}
map.put(k3, v3);
return this;
}
public AutoMatterSerializedObjectBuilder map(String k1, String v1, String k2, String v2, String k3, String v3, String k4, String v4) {
map(k1, v1, k2, v2, k3, v3);
if (k4 == null) {
throw new NullPointerException("map: k4");
}
if (v4 == null) {
throw new NullPointerException("map: v4");
}
map.put(k4, v4);
return this;
}
public AutoMatterSerializedObjectBuilder map(String k1, String v1, String k2, String v2, String k3, String v3, String k4, String v4, String k5, String v5) {
map(k1, v1, k2, v2, k3, v3, k4, v4);
if (k5 == null) {
throw new NullPointerException("map: k5");
}
if (v5 == null) {
throw new NullPointerException("map: v5");
}
map.put(k5, v5);
return this;
}
public List list() {
if (this.list == null) {
this.list = new ArrayList();
}
return list;
}
public AutoMatterSerializedObjectBuilder list(List extends String> list) {
return list((Collection extends String>) list);
}
public AutoMatterSerializedObjectBuilder list(Collection extends String> list) {
if (list == null) {
throw new NullPointerException("list");
}
for (String item : list) {
if (item == null) {
throw new NullPointerException("list: null item");
}
}
this.list = new ArrayList(list);
return this;
}
public AutoMatterSerializedObjectBuilder list(Iterable extends String> list) {
if (list == null) {
throw new NullPointerException("list");
}
if (list instanceof Collection) {
return list((Collection extends String>) list);
}
return list(list.iterator());
}
public AutoMatterSerializedObjectBuilder list(Iterator extends String> list) {
if (list == null) {
throw new NullPointerException("list");
}
this.list = new ArrayList();
while (list.hasNext()) {
String item = list.next();
if (item == null) {
throw new NullPointerException("list: null item");
}
this.list.add(item);
}
return this;
}
public AutoMatterSerializedObjectBuilder list(String... list) {
if (list == null) {
throw new NullPointerException("list");
}
return list(Arrays.asList(list));
}
public Map> optionalMap() {
if (this.optionalMap == null) {
this.optionalMap = new HashMap>();
}
return optionalMap;
}
public AutoMatterSerializedObjectBuilder optionalMap(Map extends String, ? extends List> optionalMap) {
if (optionalMap == null) {
throw new NullPointerException("optionalMap");
}
for (Map.Entry extends String, ? extends List> entry : optionalMap.entrySet()) {
if (entry.getKey() == null) {
throw new NullPointerException("optionalMap: null key");
}
if (entry.getValue() == null) {
throw new NullPointerException("optionalMap: null value");
}
}
this.optionalMap = new HashMap>(optionalMap);
return this;
}
public AutoMatterSerializedObjectBuilder optionalMap(String k1, List v1) {
if (k1 == null) {
throw new NullPointerException("optionalMap: k1");
}
if (v1 == null) {
throw new NullPointerException("optionalMap: v1");
}
optionalMap = new HashMap>();
optionalMap.put(k1, v1);
return this;
}
public AutoMatterSerializedObjectBuilder optionalMap(String k1, List v1, String k2, List v2) {
optionalMap(k1, v1);
if (k2 == null) {
throw new NullPointerException("optionalMap: k2");
}
if (v2 == null) {
throw new NullPointerException("optionalMap: v2");
}
optionalMap.put(k2, v2);
return this;
}
public AutoMatterSerializedObjectBuilder optionalMap(String k1, List v1, String k2, List v2, String k3, List v3) {
optionalMap(k1, v1, k2, v2);
if (k3 == null) {
throw new NullPointerException("optionalMap: k3");
}
if (v3 == null) {
throw new NullPointerException("optionalMap: v3");
}
optionalMap.put(k3, v3);
return this;
}
public AutoMatterSerializedObjectBuilder optionalMap(String k1, List v1, String k2, List v2, String k3, List v3, String k4, List v4) {
optionalMap(k1, v1, k2, v2, k3, v3);
if (k4 == null) {
throw new NullPointerException("optionalMap: k4");
}
if (v4 == null) {
throw new NullPointerException("optionalMap: v4");
}
optionalMap.put(k4, v4);
return this;
}
public AutoMatterSerializedObjectBuilder optionalMap(String k1, List v1, String k2, List v2, String k3, List v3, String k4, List v4, String k5, List v5) {
optionalMap(k1, v1, k2, v2, k3, v3, k4, v4);
if (k5 == null) {
throw new NullPointerException("optionalMap: k5");
}
if (v5 == null) {
throw new NullPointerException("optionalMap: v5");
}
optionalMap.put(k5, v5);
return this;
}
public Set set() {
if (this.set == null) {
this.set = new HashSet();
}
return set;
}
public AutoMatterSerializedObjectBuilder set(Set extends Long> set) {
return set((Collection extends Long>) set);
}
public AutoMatterSerializedObjectBuilder set(Collection extends Long> set) {
if (set == null) {
throw new NullPointerException("set");
}
for (Long item : set) {
if (item == null) {
throw new NullPointerException("set: null item");
}
}
this.set = new HashSet(set);
return this;
}
public AutoMatterSerializedObjectBuilder set(Iterable extends Long> set) {
if (set == null) {
throw new NullPointerException("set");
}
if (set instanceof Collection) {
return set((Collection extends Long>) set);
}
return set(set.iterator());
}
public AutoMatterSerializedObjectBuilder set(Iterator extends Long> set) {
if (set == null) {
throw new NullPointerException("set");
}
this.set = new HashSet();
while (set.hasNext()) {
Long item = set.next();
if (item == null) {
throw new NullPointerException("set: null item");
}
this.set.add(item);
}
return this;
}
public AutoMatterSerializedObjectBuilder set(Long... set) {
if (set == null) {
throw new NullPointerException("set");
}
return set(Arrays.asList(set));
}
public int[][][] deeplyNested() {
return deeplyNested;
}
public AutoMatterSerializedObjectBuilder deeplyNested(int[][][] deeplyNested) {
if (deeplyNested == null) {
throw new NullPointerException("deeplyNested");
}
this.deeplyNested = deeplyNested;
return this;
}
public AutoMatterSerializedObject build() {
Map _map = (map != null) ? Collections.unmodifiableMap(new HashMap(map)) : Collections.emptyMap();
List _list = (list != null) ? Collections.unmodifiableList(new ArrayList(list)) : Collections.emptyList();
Map> _optionalMap = (optionalMap != null) ? Collections.unmodifiableMap(new HashMap>(optionalMap)) : Collections.>emptyMap();
Set _set = (set != null) ? Collections.unmodifiableSet(new HashSet(set)) : Collections.emptySet();
return new Value(version, field, _map, _list, _optionalMap, _set, deeplyNested);
}
public static AutoMatterSerializedObjectBuilder from(AutoMatterSerializedObject v) {
return new AutoMatterSerializedObjectBuilder(v);
}
public static AutoMatterSerializedObjectBuilder from(AutoMatterSerializedObjectBuilder v) {
return new AutoMatterSerializedObjectBuilder(v);
}
private static final class Value implements AutoMatterSerializedObject {
private final int version;
private final String field;
private final Map map;
private final List list;
private final Map> optionalMap;
private final Set set;
private final int[][][] deeplyNested;
private Value(@AutoMatter.Field("version") int version, @AutoMatter.Field("field") String field, @AutoMatter.Field("map") Map map, @AutoMatter.Field("list") List list, @AutoMatter.Field("optionalMap") Map> optionalMap, @AutoMatter.Field("set") Set set, @AutoMatter.Field("deeplyNested") int[][][] deeplyNested) {
if (field == null) {
throw new NullPointerException("field");
}
if (deeplyNested == null) {
throw new NullPointerException("deeplyNested");
}
this.version = version;
this.field = field;
this.map = (map != null) ? map : Collections.emptyMap();
this.list = (list != null) ? list : Collections.emptyList();
this.optionalMap = (optionalMap != null) ? optionalMap : Collections.>emptyMap();
this.set = (set != null) ? set : Collections.emptySet();
this.deeplyNested = deeplyNested;
}
@AutoMatter.Field
@Override
public int version() {
return version;
}
@AutoMatter.Field
@Override
public String field() {
return field;
}
@AutoMatter.Field
@Override
public Map map() {
return map;
}
@AutoMatter.Field
@Override
public List list() {
return list;
}
@AutoMatter.Field
@Override
public Map> optionalMap() {
return optionalMap;
}
@AutoMatter.Field
@Override
public Set set() {
return set;
}
@AutoMatter.Field
@Override
public int[][][] deeplyNested() {
return deeplyNested;
}
public AutoMatterSerializedObjectBuilder builder() {
return new AutoMatterSerializedObjectBuilder(this);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof AutoMatterSerializedObject)) {
return false;
}
final AutoMatterSerializedObject that = (AutoMatterSerializedObject) o;
if (version != that.version()) {
return false;
}
if (field != null ? !field.equals(that.field()) : that.field() != null) {
return false;
}
if (map != null ? !map.equals(that.map()) : that.map() != null) {
return false;
}
if (list != null ? !list.equals(that.list()) : that.list() != null) {
return false;
}
if (optionalMap != null ? !optionalMap.equals(that.optionalMap()) : that.optionalMap() != null) {
return false;
}
if (set != null ? !set.equals(that.set()) : that.set() != null) {
return false;
}
if (!Arrays.equals(deeplyNested, that.deeplyNested())) {
return false;
}
return true;
}
@Override
public int hashCode() {
int result = 1;
long temp;
result = 31 * result + version;
result = 31 * result + (field != null ? field.hashCode() : 0);
result = 31 * result + (map != null ? map.hashCode() : 0);
result = 31 * result + (list != null ? list.hashCode() : 0);
result = 31 * result + (optionalMap != null ? optionalMap.hashCode() : 0);
result = 31 * result + (set != null ? set.hashCode() : 0);
result = 31 * result + (deeplyNested != null ? Arrays.hashCode(deeplyNested) : 0);
return result;
}
@Override
public String toString() {
return "AutoMatterSerializedObject{" +
"version=" + version +
", field=" + field +
", map=" + map +
", list=" + list +
", optionalMap=" + optionalMap +
", set=" + set +
", deeplyNested=" + Arrays.toString(deeplyNested) +
'}';
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy