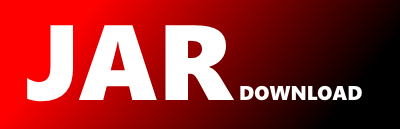
eu.toolchain.serializer.processor.annotation.AnnotationValues Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tiny-serializer-processor Show documentation
Show all versions of tiny-serializer-processor Show documentation
A processor for the @AutoSerialize annotation
The newest version!
package eu.toolchain.serializer.processor.annotation;
import static eu.toolchain.serializer.processor.Exceptions.brokenValue;
import com.google.common.collect.ImmutableList;
import java.util.List;
import java.util.Map;
import javax.lang.model.element.AnnotationMirror;
import javax.lang.model.element.AnnotationValue;
import javax.lang.model.element.Element;
import javax.lang.model.type.ErrorType;
import javax.lang.model.type.TypeMirror;
import javax.lang.model.util.SimpleAnnotationValueVisitor8;
import lombok.Data;
import lombok.RequiredArgsConstructor;
@RequiredArgsConstructor
public class AnnotationValues {
private final Element element;
private final AnnotationMirror annotation;
private final Map values;
public AnnotationValue get(final String key) {
final AnnotationValue annotationValue = values.get(key);
if (annotationValue == null) {
throw new IllegalArgumentException(key);
}
return annotationValue;
}
public Value getBoolean(final String key) {
final AnnotationValue annotationValue = values.get(key);
if (annotationValue == null) {
throw new IllegalArgumentException(key);
}
return new Value<>(annotationValue, toBoolean(annotationValue));
}
public Value getTypeMirror(final String key) {
final AnnotationValue annotationValue = values.get(key);
if (annotationValue == null) {
throw new IllegalArgumentException(key);
}
final TypeMirror typeMirror = toTypeMirror(annotationValue);
if (typeMirror == null) {
throw brokenValue("Could not resolve type", element, annotation, annotationValue);
}
if (typeMirror instanceof ErrorType) {
throw brokenValue("Could not resolve type", element, annotation, annotationValue);
}
return new Value<>(annotationValue, typeMirror);
}
public Value getString(final String key) {
final AnnotationValue annotationValue = values.get(key);
if (annotationValue == null) {
throw new IllegalArgumentException(key);
}
return new Value<>(annotationValue, toString(annotationValue));
}
public Value getShort(String key) {
final AnnotationValue annotationValue = values.get(key);
if (annotationValue == null) {
throw new IllegalArgumentException(key);
}
return new Value<>(annotationValue, toShort(annotationValue));
}
public Value getInteger(String key) {
final AnnotationValue annotationValue = values.get(key);
if (annotationValue == null) {
throw new IllegalArgumentException(key);
}
return new Value<>(annotationValue, toInteger(annotationValue));
}
public Value> getAnnotationValue(String key) {
final AnnotationValue annotationValue = values.get(key);
if (annotationValue == null) {
throw new IllegalArgumentException(key);
}
return new Value<>(annotationValue, toAnnotationMirror(annotationValue));
}
public TypeMirror toTypeMirror(final AnnotationValue annotationValue) {
return annotationValue.accept(new SimpleAnnotationValueVisitor8() {
@Override
public TypeMirror visitType(TypeMirror t, Void p) {
return t;
}
@Override
protected TypeMirror defaultAction(Object o, Void p) {
return null;
}
}, null);
}
public String toString(final AnnotationValue annotationValue) {
return annotationValue.accept(new SimpleAnnotationValueVisitor8() {
@Override
public String visitString(String s, Void p) {
return s;
}
@Override
protected String defaultAction(Object o, Void p) {
throw new IllegalArgumentException();
}
}, null);
}
public short toShort(final AnnotationValue annotationValue) {
return annotationValue.accept(new SimpleAnnotationValueVisitor8() {
@Override
public Short visitInt(int i, Void p) {
return Integer.valueOf(i).shortValue();
}
@Override
public Short visitShort(short s, Void p) {
return s;
}
@Override
protected Short defaultAction(Object o, Void p) {
throw new IllegalArgumentException(
String.format("Could not convert %s to Short", annotationValue));
}
}, null);
}
public int toInteger(final AnnotationValue annotationValue) {
return annotationValue.accept(new SimpleAnnotationValueVisitor8() {
@Override
public Integer visitInt(int i, Void p) {
return i;
}
@Override
public Integer visitShort(short s, Void p) {
return Short.valueOf(s).intValue();
}
@Override
protected Integer defaultAction(Object o, Void p) {
throw new IllegalArgumentException(
String.format("Could not convert %s to Integer", annotationValue));
}
}, null);
}
public List toAnnotationMirror(final AnnotationValue annotationValue) {
return annotationValue.accept(
new SimpleAnnotationValueVisitor8, Void>() {
@Override
public List visitAnnotation(AnnotationMirror a, Void p) {
return ImmutableList.of(a);
}
@Override
public List visitArray(
List extends AnnotationValue> vals, Void p
) {
final ImmutableList.Builder mirrors = ImmutableList.builder();
for (final AnnotationValue val : vals) {
mirrors.add(val.accept(new SimpleAnnotationValueVisitor8() {
public AnnotationMirror visitAnnotation(
AnnotationMirror a, Void p
) {
return a;
}
;
@Override
protected AnnotationMirror defaultAction(Object o, Void p) {
throw new IllegalArgumentException(
String.format("Could not convert %s to AnnotationMirror", annotationValue));
}
}, null));
}
return mirrors.build();
}
@Override
protected List defaultAction(Object o, Void p) {
throw new IllegalArgumentException(
String.format("Could not convert %s to AnnotationMirror", annotationValue));
}
}, null);
}
public boolean toBoolean(AnnotationValue annotationValue) {
return annotationValue.accept(new SimpleAnnotationValueVisitor8() {
@Override
public Boolean visitBoolean(boolean b, Void p) {
return b;
}
@Override
protected Boolean defaultAction(Object o, Void p) {
throw new IllegalArgumentException(
String.format("Could not convert %s to Boolean", annotationValue));
}
}, null);
}
@Data
public static class Value {
private final AnnotationValue annotationValue;
private final T value;
public T get() {
return value;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy