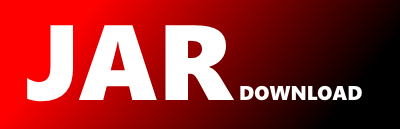
eu.toolchain.serializer.processor.field.FieldBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tiny-serializer-processor Show documentation
Show all versions of tiny-serializer-processor Show documentation
A processor for the @AutoSerialize annotation
The newest version!
package eu.toolchain.serializer.processor.field;
import com.google.common.base.CaseFormat;
import com.google.common.base.Joiner;
import com.google.common.collect.ImmutableList;
import com.squareup.javapoet.ClassName;
import com.squareup.javapoet.MethodSpec;
import com.squareup.javapoet.TypeName;
import eu.toolchain.serializer.processor.annotation.BuilderMirror;
import java.util.List;
import lombok.Data;
/**
* @author udoprog
*/
@Data
public class FieldBuilder {
static final Joiner emptyJoiner = Joiner.on("");
final BuilderMirror builder;
/**
* If {@code true}, indicates that the constructor of the builder should be used when
* instantiating it.
*/
final boolean useConstructor;
/**
* If {@code true}, indicates that builder methods are setters instead of using a one-to-one
* mapping of field names.
*/
final boolean useSetter;
/**
* Contains the name of the method to use, unless {@link #useConstructor} is {@code true}.
*/
final String method;
public void writeTo(ClassName returnType, MethodSpec.Builder b, List variables) {
final ImmutableList.Builder builders = ImmutableList.builder();
final ImmutableList.Builder
© 2015 - 2025 Weber Informatics LLC | Privacy Policy