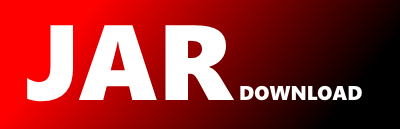
eu.toop.edm.jaxb.dcatap.ObjectFactory Maven / Gradle / Ivy
package eu.toop.edm.jaxb.dcatap;
import java.math.BigDecimal;
import java.time.Duration;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import javax.xml.namespace.QName;
import com.helger.commons.annotation.CodingStyleguideUnaware;
import com.helger.jaxb.adapter.AdapterDuration;
import eu.toop.edm.jaxb.dcterms.DCMediaType;
import eu.toop.edm.jaxb.foaf.FoafDocumentType;
import eu.toop.edm.jaxb.rdf.PlainLiteral;
import eu.toop.edm.jaxb.w3.skos.ConceptSchemeType;
import eu.toop.edm.jaxb.w3.skos.ConceptType;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the eu.toop.edm.jaxb.dcatap package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
This class was annotated by ph-jaxb22-plugin -Xph-annotate
*
*
*/
@XmlRegistry
@CodingStyleguideUnaware
public class ObjectFactory {
public final static QName _AccessURL_QNAME = new QName("http://data.europa.eu/r5r/", "accessURL");
public final static QName _ByteSize_QNAME = new QName("http://data.europa.eu/r5r/", "byteSize");
public final static QName _DownloadURL_QNAME = new QName("http://data.europa.eu/r5r/", "downloadURL");
public final static QName _EndpointURL_QNAME = new QName("http://data.europa.eu/r5r/", "endpointURL");
public final static QName _EndpointDescription_QNAME = new QName("http://data.europa.eu/r5r/", "endpointDescription");
public final static QName _HadRole_QNAME = new QName("http://data.europa.eu/r5r/", "hadRole");
public final static QName _SpatialResolutionInMeters_QNAME = new QName("http://data.europa.eu/r5r/", "spatialResolutionInMeters");
public final static QName _TemporalResolution_QNAME = new QName("http://data.europa.eu/r5r/", "temporalResolution");
public final static QName _AccessService_QNAME = new QName("http://data.europa.eu/r5r/", "accessService");
public final static QName _Availability_QNAME = new QName("http://data.europa.eu/r5r/", "availability");
public final static QName _Catalog_QNAME = new QName("http://data.europa.eu/r5r/", "catalog");
public final static QName _CompressedFormat_QNAME = new QName("http://data.europa.eu/r5r/", "compressedFormat");
public final static QName _Dataset_QNAME = new QName("http://data.europa.eu/r5r/", "dataset");
public final static QName _HasPart_QNAME = new QName("http://data.europa.eu/r5r/", "hasPart");
public final static QName _HasVersion_QNAME = new QName("http://data.europa.eu/r5r/", "hasVersion");
public final static QName _IsPartOf_QNAME = new QName("http://data.europa.eu/r5r/", "isPartOf");
public final static QName _IsVersionOf_QNAME = new QName("http://data.europa.eu/r5r/", "isVersionOf");
public final static QName _Record_QNAME = new QName("http://data.europa.eu/r5r/", "record");
public final static QName _ThemeTaxonomy_QNAME = new QName("http://data.europa.eu/r5r/", "themeTaxonomy");
public final static QName _Service_QNAME = new QName("http://data.europa.eu/r5r/", "service");
public final static QName _ContactPoint_QNAME = new QName("http://data.europa.eu/r5r/", "contactPoint");
public final static QName _Distribution_QNAME = new QName("http://data.europa.eu/r5r/", "distribution");
public final static QName _Keyword_QNAME = new QName("http://data.europa.eu/r5r/", "keyword");
public final static QName _LandingPage_QNAME = new QName("http://data.europa.eu/r5r/", "landingPage");
public final static QName _MediaType_QNAME = new QName("http://data.europa.eu/r5r/", "mediaType");
public final static QName _PackageFormat_QNAME = new QName("http://data.europa.eu/r5r/", "packageFormat");
public final static QName _QualifiedRelation_QNAME = new QName("http://data.europa.eu/r5r/", "qualifiedRelation");
public final static QName _ServesDataset_QNAME = new QName("http://data.europa.eu/r5r/", "servesDataset");
public final static QName _Source_QNAME = new QName("http://data.europa.eu/r5r/", "source");
public final static QName _Theme_QNAME = new QName("http://data.europa.eu/r5r/", "theme");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: eu.toop.edm.jaxb.dcatap
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link DCatAPCatalogRecordType }
*
* @return
* The created DCatAPCatalogRecordType object and never null
.
*/
@Nonnull
public DCatAPCatalogRecordType createDCatAPCatalogRecordType() {
return new DCatAPCatalogRecordType();
}
/**
* Create an instance of {@link DCatAPDistributionType }
*
* @return
* The created DCatAPDistributionType object and never null
.
*/
@Nonnull
public DCatAPDistributionType createDCatAPDistributionType() {
return new DCatAPDistributionType();
}
/**
* Create an instance of {@link DCatAPRoleType }
*
* @return
* The created DCatAPRoleType object and never null
.
*/
@Nonnull
public DCatAPRoleType createDCatAPRoleType() {
return new DCatAPRoleType();
}
/**
* Create an instance of {@link DCatAPDataServiceType }
*
* @return
* The created DCatAPDataServiceType object and never null
.
*/
@Nonnull
public DCatAPDataServiceType createDCatAPDataServiceType() {
return new DCatAPDataServiceType();
}
/**
* Create an instance of {@link DCatAPCatalogType }
*
* @return
* The created DCatAPCatalogType object and never null
.
*/
@Nonnull
public DCatAPCatalogType createDCatAPCatalogType() {
return new DCatAPCatalogType();
}
/**
* Create an instance of {@link DCatAPDatasetType }
*
* @return
* The created DCatAPDatasetType object and never null
.
*/
@Nonnull
public DCatAPDatasetType createDCatAPDatasetType() {
return new DCatAPDatasetType();
}
/**
* Create an instance of {@link DCatAPRelationshipType }
*
* @return
* The created DCatAPRelationshipType object and never null
.
*/
@Nonnull
public DCatAPRelationshipType createDCatAPRelationshipType() {
return new DCatAPRelationshipType();
}
/**
* Create an instance of {@link DCatAPCatalogRecordType.CatalogRecord }
*
* @return
* The created CatalogRecord object and never null
.
*/
@Nonnull
public DCatAPCatalogRecordType.CatalogRecord createDCatAPCatalogRecordTypeCatalogRecord() {
return new DCatAPCatalogRecordType.CatalogRecord();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://data.europa.eu/r5r/", name = "accessURL")
@Nonnull
public JAXBElement createAccessURL(
@Nullable
final String value) {
return new JAXBElement(_AccessURL_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://data.europa.eu/r5r/", name = "byteSize")
@Nonnull
public JAXBElement createByteSize(
@Nullable
final BigDecimal value) {
return new JAXBElement(_ByteSize_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://data.europa.eu/r5r/", name = "downloadURL")
@Nonnull
public JAXBElement createDownloadURL(
@Nullable
final String value) {
return new JAXBElement(_DownloadURL_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://data.europa.eu/r5r/", name = "endpointURL")
@Nonnull
public JAXBElement createEndpointURL(
@Nullable
final String value) {
return new JAXBElement(_EndpointURL_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://data.europa.eu/r5r/", name = "endpointDescription")
@Nonnull
public JAXBElement createEndpointDescription(
@Nullable
final String value) {
return new JAXBElement(_EndpointDescription_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link DCatAPRoleType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link DCatAPRoleType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://data.europa.eu/r5r/", name = "hadRole")
@Nonnull
public JAXBElement createHadRole(
@Nullable
final DCatAPRoleType value) {
return new JAXBElement(_HadRole_QNAME, DCatAPRoleType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://data.europa.eu/r5r/", name = "spatialResolutionInMeters")
@Nonnull
public JAXBElement createSpatialResolutionInMeters(
@Nullable
final BigDecimal value) {
return new JAXBElement(_SpatialResolutionInMeters_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Duration }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Duration }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://data.europa.eu/r5r/", name = "temporalResolution")
@XmlJavaTypeAdapter(AdapterDuration.class)
@Nonnull
public JAXBElement createTemporalResolution(
@Nullable
final Duration value) {
return new JAXBElement(_TemporalResolution_QNAME, Duration.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link DCatAPDataServiceType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link DCatAPDataServiceType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://data.europa.eu/r5r/", name = "accessService")
@Nonnull
public JAXBElement createAccessService(
@Nullable
final DCatAPDataServiceType value) {
return new JAXBElement(_AccessService_QNAME, DCatAPDataServiceType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ConceptType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ConceptType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://data.europa.eu/r5r/", name = "availability")
@Nonnull
public JAXBElement createAvailability(
@Nullable
final ConceptType value) {
return new JAXBElement(_Availability_QNAME, ConceptType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link DCatAPCatalogType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link DCatAPCatalogType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://data.europa.eu/r5r/", name = "catalog")
@Nonnull
public JAXBElement createCatalog(
@Nullable
final DCatAPCatalogType value) {
return new JAXBElement(_Catalog_QNAME, DCatAPCatalogType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link DCMediaType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link DCMediaType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://data.europa.eu/r5r/", name = "compressedFormat")
@Nonnull
public JAXBElement createCompressedFormat(
@Nullable
final DCMediaType value) {
return new JAXBElement(_CompressedFormat_QNAME, DCMediaType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link DCatAPDatasetType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link DCatAPDatasetType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://data.europa.eu/r5r/", name = "dataset")
@Nonnull
public JAXBElement createDataset(
@Nullable
final DCatAPDatasetType value) {
return new JAXBElement(_Dataset_QNAME, DCatAPDatasetType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link DCatAPCatalogType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link DCatAPCatalogType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://data.europa.eu/r5r/", name = "hasPart")
@Nonnull
public JAXBElement createHasPart(
@Nullable
final DCatAPCatalogType value) {
return new JAXBElement(_HasPart_QNAME, DCatAPCatalogType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link DCatAPDatasetType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link DCatAPDatasetType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://data.europa.eu/r5r/", name = "hasVersion")
@Nonnull
public JAXBElement createHasVersion(
@Nullable
final DCatAPDatasetType value) {
return new JAXBElement(_HasVersion_QNAME, DCatAPDatasetType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link DCatAPCatalogType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link DCatAPCatalogType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://data.europa.eu/r5r/", name = "isPartOf")
@Nonnull
public JAXBElement createIsPartOf(
@Nullable
final DCatAPCatalogType value) {
return new JAXBElement(_IsPartOf_QNAME, DCatAPCatalogType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link DCatAPDatasetType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link DCatAPDatasetType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://data.europa.eu/r5r/", name = "isVersionOf")
@Nonnull
public JAXBElement createIsVersionOf(
@Nullable
final DCatAPDatasetType value) {
return new JAXBElement(_IsVersionOf_QNAME, DCatAPDatasetType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link DCatAPCatalogRecordType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link DCatAPCatalogRecordType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://data.europa.eu/r5r/", name = "record")
@Nonnull
public JAXBElement createRecord(
@Nullable
final DCatAPCatalogRecordType value) {
return new JAXBElement(_Record_QNAME, DCatAPCatalogRecordType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ConceptSchemeType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ConceptSchemeType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://data.europa.eu/r5r/", name = "themeTaxonomy")
@Nonnull
public JAXBElement createThemeTaxonomy(
@Nullable
final ConceptSchemeType value) {
return new JAXBElement(_ThemeTaxonomy_QNAME, ConceptSchemeType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link DCatAPDataServiceType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link DCatAPDataServiceType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://data.europa.eu/r5r/", name = "service")
@Nonnull
public JAXBElement createService(
@Nullable
final DCatAPDataServiceType value) {
return new JAXBElement(_Service_QNAME, DCatAPDataServiceType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Object }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Object }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://data.europa.eu/r5r/", name = "contactPoint")
@Nonnull
public JAXBElement
© 2015 - 2025 Weber Informatics LLC | Privacy Policy