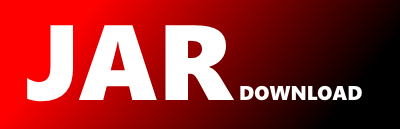
eu.toop.edm.jaxb.dcterms.ObjectFactory Maven / Gradle / Ivy
package eu.toop.edm.jaxb.dcterms;
import java.math.BigInteger;
import java.time.LocalDate;
import java.time.LocalDateTime;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.bind.annotation.adapters.NormalizedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import javax.xml.namespace.QName;
import com.helger.commons.annotation.CodingStyleguideUnaware;
import com.helger.jaxb.adapter.AdapterLocalDate;
import com.helger.jaxb.adapter.AdapterLocalDateTime;
import eu.toop.edm.jaxb.foaf.FoafAgentType;
import eu.toop.edm.jaxb.rdf.Resource;
import eu.toop.edm.jaxb.w3.skos.ConceptType;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the eu.toop.edm.jaxb.dcterms package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
This class was annotated by ph-jaxb22-plugin -Xph-annotate
*
*
*/
@XmlRegistry
@CodingStyleguideUnaware
public class ObjectFactory {
public final static QName _Description_QNAME = new QName("http://purl.org/dc/terms/", "description");
public final static QName _EndDate_QNAME = new QName("http://purl.org/dc/terms/", "endDate");
public final static QName _Identifier_QNAME = new QName("http://purl.org/dc/terms/", "identifier");
public final static QName _Issued_QNAME = new QName("http://purl.org/dc/terms/", "issued");
public final static QName _Modified_QNAME = new QName("http://purl.org/dc/terms/", "modified");
public final static QName _Publisher_QNAME = new QName("http://purl.org/dc/terms/", "publisher");
public final static QName _StartDate_QNAME = new QName("http://purl.org/dc/terms/", "startDate");
public final static QName _Title_QNAME = new QName("http://purl.org/dc/terms/", "title");
public final static QName _Type_QNAME = new QName("http://purl.org/dc/terms/", "type");
public final static QName _AccessRights_QNAME = new QName("http://purl.org/dc/terms/", "accessRights");
public final static QName _AccrualPeriodicity_QNAME = new QName("http://purl.org/dc/terms/", "accrualPeriodicity");
public final static QName _ConformsTo_QNAME = new QName("http://purl.org/dc/terms/", "conformsTo");
public final static QName _Creator_QNAME = new QName("http://purl.org/dc/terms/", "creator");
public final static QName _Format_QNAME = new QName("http://purl.org/dc/terms/", "format");
public final static QName _HasVersion_QNAME = new QName("http://purl.org/dc/terms/", "hasVersion");
public final static QName _IsReferencedBy_QNAME = new QName("http://purl.org/dc/terms/", "isReferencedBy");
public final static QName _IsVersionOf_QNAME = new QName("http://purl.org/dc/terms/", "isVersionOf");
public final static QName _Licence_QNAME = new QName("http://purl.org/dc/terms/", "licence");
public final static QName _Language_QNAME = new QName("http://purl.org/dc/terms/", "language");
public final static QName _Provenance_QNAME = new QName("http://purl.org/dc/terms/", "provenance");
public final static QName _Relation_QNAME = new QName("http://purl.org/dc/terms/", "relation");
public final static QName _Rights_QNAME = new QName("http://purl.org/dc/terms/", "rights");
public final static QName _Source_QNAME = new QName("http://purl.org/dc/terms/", "source");
public final static QName _Spatial_QNAME = new QName("http://purl.org/dc/terms/", "spatial");
public final static QName _Temporal_QNAME = new QName("http://purl.org/dc/terms/", "temporal");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: eu.toop.edm.jaxb.dcterms
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link DCMediaType }
*
* @return
* The created DCMediaType object and never null
.
*/
@Nonnull
public DCMediaType createDCMediaType() {
return new DCMediaType();
}
/**
* Create an instance of {@link DCRightStatementType }
*
* @return
* The created DCRightStatementType object and never null
.
*/
@Nonnull
public DCRightStatementType createDCRightStatementType() {
return new DCRightStatementType();
}
/**
* Create an instance of {@link DCStandardType }
*
* @return
* The created DCStandardType object and never null
.
*/
@Nonnull
public DCStandardType createDCStandardType() {
return new DCStandardType();
}
/**
* Create an instance of {@link DCLicenceDocumentType }
*
* @return
* The created DCLicenceDocumentType object and never null
.
*/
@Nonnull
public DCLicenceDocumentType createDCLicenceDocumentType() {
return new DCLicenceDocumentType();
}
/**
* Create an instance of {@link DCProvenanceStatementType }
*
* @return
* The created DCProvenanceStatementType object and never null
.
*/
@Nonnull
public DCProvenanceStatementType createDCProvenanceStatementType() {
return new DCProvenanceStatementType();
}
/**
* Create an instance of {@link DCLocationType }
*
* @return
* The created DCLocationType object and never null
.
*/
@Nonnull
public DCLocationType createDCLocationType() {
return new DCLocationType();
}
/**
* Create an instance of {@link DCPeriodOfTimeType }
*
* @return
* The created DCPeriodOfTimeType object and never null
.
*/
@Nonnull
public DCPeriodOfTimeType createDCPeriodOfTimeType() {
return new DCPeriodOfTimeType();
}
/**
* Create an instance of {@link DCCodeType }
*
* @return
* The created DCCodeType object and never null
.
*/
@Nonnull
public DCCodeType createDCCodeType() {
return new DCCodeType();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://purl.org/dc/terms/", name = "description")
@Nonnull
public JAXBElement createDescription(
@Nullable
final String value) {
return new JAXBElement(_Description_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://purl.org/dc/terms/", name = "endDate")
@XmlJavaTypeAdapter(AdapterLocalDate.class)
@Nonnull
public JAXBElement createEndDate(
@Nullable
final LocalDate value) {
return new JAXBElement(_EndDate_QNAME, LocalDate.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://purl.org/dc/terms/", name = "identifier")
@XmlJavaTypeAdapter(NormalizedStringAdapter.class)
@Nonnull
public JAXBElement createIdentifier(
@Nullable
final String value) {
return new JAXBElement(_Identifier_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocalDateTime }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocalDateTime }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://purl.org/dc/terms/", name = "issued")
@XmlJavaTypeAdapter(AdapterLocalDateTime.class)
@Nonnull
public JAXBElement createIssued(
@Nullable
final LocalDateTime value) {
return new JAXBElement(_Issued_QNAME, LocalDateTime.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocalDateTime }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocalDateTime }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://purl.org/dc/terms/", name = "modified")
@XmlJavaTypeAdapter(AdapterLocalDateTime.class)
@Nonnull
public JAXBElement createModified(
@Nullable
final LocalDateTime value) {
return new JAXBElement(_Modified_QNAME, LocalDateTime.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link FoafAgentType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link FoafAgentType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://purl.org/dc/terms/", name = "publisher")
@Nonnull
public JAXBElement createPublisher(
@Nullable
final FoafAgentType value) {
return new JAXBElement(_Publisher_QNAME, FoafAgentType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://purl.org/dc/terms/", name = "startDate")
@XmlJavaTypeAdapter(AdapterLocalDate.class)
@Nonnull
public JAXBElement createStartDate(
@Nullable
final LocalDate value) {
return new JAXBElement(_StartDate_QNAME, LocalDate.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://purl.org/dc/terms/", name = "title")
@Nonnull
public JAXBElement createTitle(
@Nullable
final String value) {
return new JAXBElement(_Title_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://purl.org/dc/terms/", name = "type")
@Nonnull
public JAXBElement createType(
@Nullable
final String value) {
return new JAXBElement(_Type_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link DCRightStatementType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link DCRightStatementType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://purl.org/dc/terms/", name = "accessRights")
@Nonnull
public JAXBElement createAccessRights(
@Nullable
final DCRightStatementType value) {
return new JAXBElement(_AccessRights_QNAME, DCRightStatementType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigInteger }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigInteger }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://purl.org/dc/terms/", name = "accrualPeriodicity")
@Nonnull
public JAXBElement createAccrualPeriodicity(
@Nullable
final BigInteger value) {
return new JAXBElement(_AccrualPeriodicity_QNAME, BigInteger.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link DCStandardType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link DCStandardType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://purl.org/dc/terms/", name = "conformsTo")
@Nonnull
public JAXBElement createConformsTo(
@Nullable
final DCStandardType value) {
return new JAXBElement(_ConformsTo_QNAME, DCStandardType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link FoafAgentType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link FoafAgentType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://purl.org/dc/terms/", name = "creator")
@Nonnull
public JAXBElement createCreator(
@Nullable
final FoafAgentType value) {
return new JAXBElement(_Creator_QNAME, FoafAgentType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link DCMediaType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link DCMediaType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://purl.org/dc/terms/", name = "format")
@Nonnull
public JAXBElement createFormat(
@Nullable
final DCMediaType value) {
return new JAXBElement(_Format_QNAME, DCMediaType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Resource }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Resource }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://purl.org/dc/terms/", name = "hasVersion")
@Nonnull
public JAXBElement createHasVersion(
@Nullable
final Resource value) {
return new JAXBElement(_HasVersion_QNAME, Resource.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Resource }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Resource }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://purl.org/dc/terms/", name = "isReferencedBy")
@Nonnull
public JAXBElement createIsReferencedBy(
@Nullable
final Resource value) {
return new JAXBElement(_IsReferencedBy_QNAME, Resource.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Resource }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Resource }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://purl.org/dc/terms/", name = "isVersionOf")
@Nonnull
public JAXBElement createIsVersionOf(
@Nullable
final Resource value) {
return new JAXBElement(_IsVersionOf_QNAME, Resource.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link DCLicenceDocumentType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link DCLicenceDocumentType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://purl.org/dc/terms/", name = "licence")
@Nonnull
public JAXBElement createLicence(
@Nullable
final DCLicenceDocumentType value) {
return new JAXBElement(_Licence_QNAME, DCLicenceDocumentType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://purl.org/dc/terms/", name = "language")
@Nonnull
public JAXBElement createLanguage(
@Nullable
final String value) {
return new JAXBElement(_Language_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link DCProvenanceStatementType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link DCProvenanceStatementType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://purl.org/dc/terms/", name = "provenance")
@Nonnull
public JAXBElement createProvenance(
@Nullable
final DCProvenanceStatementType value) {
return new JAXBElement(_Provenance_QNAME, DCProvenanceStatementType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Object }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Object }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://purl.org/dc/terms/", name = "relation")
@Nonnull
public JAXBElement
© 2015 - 2025 Weber Informatics LLC | Privacy Policy