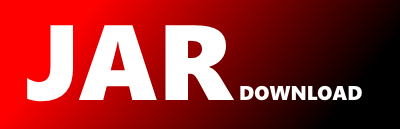
eu.toop.edm.jaxb.prov.ObjectFactory Maven / Gradle / Ivy
package eu.toop.edm.jaxb.prov;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.namespace.QName;
import com.helger.commons.annotation.CodingStyleguideUnaware;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the eu.toop.edm.jaxb.prov package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
This class was annotated by ph-jaxb22-plugin -Xph-annotate
*
*
*/
@XmlRegistry
@CodingStyleguideUnaware
public class ObjectFactory {
public final static QName _ActedOnBehalfOf_QNAME = new QName("http://www.w3.org/ns/prov#", "actedOnBehalfOf");
public final static QName _Activity_QNAME = new QName("http://www.w3.org/ns/prov#", "activity");
public final static QName _Agent_QNAME = new QName("http://www.w3.org/ns/prov#", "agent");
public final static QName _AtLocation_QNAME = new QName("http://www.w3.org/ns/prov#", "atLocation");
public final static QName _Entity_QNAME = new QName("http://www.w3.org/ns/prov#", "entity");
public final static QName _HadActivity_QNAME = new QName("http://www.w3.org/ns/prov#", "hadActivity");
public final static QName _HadPlan_QNAME = new QName("http://www.w3.org/ns/prov#", "hadPlan");
public final static QName _HadRole_QNAME = new QName("http://www.w3.org/ns/prov#", "hadRole");
public final static QName _QualifiedAttribution_QNAME = new QName("http://www.w3.org/ns/prov#", "qualifiedAttribution");
public final static QName _QualifiedAssotiation_QNAME = new QName("http://www.w3.org/ns/prov#", "qualifiedAssotiation");
public final static QName _QualifiedInfluence_QNAME = new QName("http://www.w3.org/ns/prov#", "qualifiedInfluence");
public final static QName _WasInfluencedBy_QNAME = new QName("http://www.w3.org/ns/prov#", "wasInfluencedBy");
public final static QName _WasGeneratedBy_QNAME = new QName("http://www.w3.org/ns/prov#", "wasGeneratedBy");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: eu.toop.edm.jaxb.prov
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link AgentType }
*
* @return
* The created AgentType object and never null
.
*/
@Nonnull
public AgentType createAgentType() {
return new AgentType();
}
/**
* Create an instance of {@link ActivityType }
*
* @return
* The created ActivityType object and never null
.
*/
@Nonnull
public ActivityType createActivityType() {
return new ActivityType();
}
/**
* Create an instance of {@link AttributionType }
*
* @return
* The created AttributionType object and never null
.
*/
@Nonnull
public AttributionType createAttributionType() {
return new AttributionType();
}
/**
* Create an instance of {@link AssotiationType }
*
* @return
* The created AssotiationType object and never null
.
*/
@Nonnull
public AssotiationType createAssotiationType() {
return new AssotiationType();
}
/**
* Create an instance of {@link InfluenceType }
*
* @return
* The created InfluenceType object and never null
.
*/
@Nonnull
public InfluenceType createInfluenceType() {
return new InfluenceType();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AgentType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link AgentType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.w3.org/ns/prov#", name = "actedOnBehalfOf")
@Nonnull
public JAXBElement createActedOnBehalfOf(
@Nullable
final AgentType value) {
return new JAXBElement(_ActedOnBehalfOf_QNAME, AgentType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ActivityType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ActivityType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.w3.org/ns/prov#", name = "activity")
@Nonnull
public JAXBElement createActivity(
@Nullable
final ActivityType value) {
return new JAXBElement(_Activity_QNAME, ActivityType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AgentType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link AgentType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.w3.org/ns/prov#", name = "agent")
@Nonnull
public JAXBElement createAgent(
@Nullable
final AgentType value) {
return new JAXBElement(_Agent_QNAME, AgentType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Object }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Object }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.w3.org/ns/prov#", name = "atLocation")
@Nonnull
public JAXBElement
© 2015 - 2025 Weber Informatics LLC | Privacy Policy