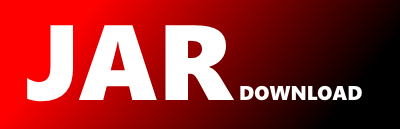
eu.toop.edm.jaxb.vcard.ObjectFactory Maven / Gradle / Ivy
package eu.toop.edm.jaxb.vcard;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.namespace.QName;
import com.helger.commons.annotation.CodingStyleguideUnaware;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the eu.toop.edm.jaxb.vcard package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
This class was annotated by ph-jaxb22-plugin -Xph-annotate
*
*
*/
@XmlRegistry
@CodingStyleguideUnaware
public class ObjectFactory {
public final static QName _VCard_QNAME = new QName("http://www.w3.org/2001/vcard-rdf/3.0#", "VCard");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: eu.toop.edm.jaxb.vcard
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link VCARDType }
*
* @return
* The created VCARDType object and never null
.
*/
@Nonnull
public VCARDType createVCARDType() {
return new VCARDType();
}
/**
* Create an instance of {@link SimpleElement }
*
* @return
* The created SimpleElement object and never null
.
*/
@Nonnull
public SimpleElement createSimpleElement() {
return new SimpleElement();
}
/**
* Create an instance of {@link BinaryElement }
*
* @return
* The created BinaryElement object and never null
.
*/
@Nonnull
public BinaryElement createBinaryElement() {
return new BinaryElement();
}
/**
* Create an instance of {@link VCARDType.VCARD }
*
* @return
* The created VCARD object and never null
.
*/
@Nonnull
public VCARDType.VCARD createVCARDTypeVCARD() {
return new VCARDType.VCARD();
}
/**
* Create an instance of {@link VCARDType.TEL }
*
* @return
* The created TEL object and never null
.
*/
@Nonnull
public VCARDType.TEL createVCARDTypeTEL() {
return new VCARDType.TEL();
}
/**
* Create an instance of {@link VCARDType.EMAIL }
*
* @return
* The created EMAIL object and never null
.
*/
@Nonnull
public VCARDType.EMAIL createVCARDTypeEMAIL() {
return new VCARDType.EMAIL();
}
/**
* Create an instance of {@link VCARDType.ADR }
*
* @return
* The created ADR object and never null
.
*/
@Nonnull
public VCARDType.ADR createVCARDTypeADR() {
return new VCARDType.ADR();
}
/**
* Create an instance of {@link VCARDType.ORG }
*
* @return
* The created ORG object and never null
.
*/
@Nonnull
public VCARDType.ORG createVCARDTypeORG() {
return new VCARDType.ORG();
}
/**
* Create an instance of {@link VCARDType.N }
*
* @return
* The created N object and never null
.
*/
@Nonnull
public VCARDType.N createVCARDTypeN() {
return new VCARDType.N();
}
/**
* Create an instance of {@link VCARDType.LABEL }
*
* @return
* The created LABEL object and never null
.
*/
@Nonnull
public VCARDType.LABEL createVCARDTypeLABEL() {
return new VCARDType.LABEL();
}
/**
* Create an instance of {@link VCARDType.TZ }
*
* @return
* The created TZ object and never null
.
*/
@Nonnull
public VCARDType.TZ createVCARDTypeTZ() {
return new VCARDType.TZ();
}
/**
* Create an instance of {@link VCARDType.UID }
*
* @return
* The created UID object and never null
.
*/
@Nonnull
public VCARDType.UID createVCARDTypeUID() {
return new VCARDType.UID();
}
/**
* Create an instance of {@link VCARDType.AGENT }
*
* @return
* The created AGENT object and never null
.
*/
@Nonnull
public VCARDType.AGENT createVCARDTypeAGENT() {
return new VCARDType.AGENT();
}
/**
* Create an instance of {@link VCARDType.GROUP }
*
* @return
* The created GROUP object and never null
.
*/
@Nonnull
public VCARDType.GROUP createVCARDTypeGROUP() {
return new VCARDType.GROUP();
}
/**
* Create an instance of {@link VCARDType.URL }
*
* @return
* The created URL object and never null
.
*/
@Nonnull
public VCARDType.URL createVCARDTypeURL() {
return new VCARDType.URL();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link VCARDType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link VCARDType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.w3.org/2001/vcard-rdf/3.0#", name = "VCard")
@Nonnull
public JAXBElement createVCard(
@Nullable
final VCARDType value) {
return new JAXBElement(_VCard_QNAME, VCARDType.class, null, value);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy