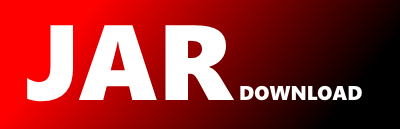
eu.toop.edm.jaxb.w3.locn.ObjectFactory Maven / Gradle / Ivy
package eu.toop.edm.jaxb.w3.locn;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.namespace.QName;
import com.helger.commons.annotation.CodingStyleguideUnaware;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the eu.toop.edm.jaxb.w3.locn package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
This class was annotated by ph-jaxb22-plugin -Xph-annotate
*
*
*/
@XmlRegistry
@CodingStyleguideUnaware
public class ObjectFactory {
public final static QName _PoBox_QNAME = new QName("http://www.w3.org/ns/locn#", "poBox");
public final static QName _Thoroughfare_QNAME = new QName("http://www.w3.org/ns/locn#", "thoroughfare");
public final static QName _LocatorDesignator_QNAME = new QName("http://www.w3.org/ns/locn#", "locatorDesignator");
public final static QName _LocatorName_QNAME = new QName("http://www.w3.org/ns/locn#", "locatorName");
public final static QName _AddressArea_QNAME = new QName("http://www.w3.org/ns/locn#", "addressArea");
public final static QName _PostName_QNAME = new QName("http://www.w3.org/ns/locn#", "postName");
public final static QName _AdminUnitLevel1_QNAME = new QName("http://www.w3.org/ns/locn#", "adminUnitLevel1");
public final static QName _AdminUnitLevel2_QNAME = new QName("http://www.w3.org/ns/locn#", "adminUnitLevel2");
public final static QName _PostCode_QNAME = new QName("http://www.w3.org/ns/locn#", "postCode");
public final static QName _AddressId_QNAME = new QName("http://www.w3.org/ns/locn#", "addressId");
public final static QName _Address_QNAME = new QName("http://www.w3.org/ns/locn#", "address");
public final static QName _Geometry_QNAME = new QName("http://www.w3.org/ns/locn#", "Geometry");
public final static QName _Location_QNAME = new QName("http://www.w3.org/ns/locn#", "Location");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: eu.toop.edm.jaxb.w3.locn
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link AddressType }
*
* @return
* The created AddressType object and never null
.
*/
@Nonnull
public AddressType createAddressType() {
return new AddressType();
}
/**
* Create an instance of {@link GeometryType }
*
* @return
* The created GeometryType object and never null
.
*/
@Nonnull
public GeometryType createGeometryType() {
return new GeometryType();
}
/**
* Create an instance of {@link LocationType }
*
* @return
* The created LocationType object and never null
.
*/
@Nonnull
public LocationType createLocationType() {
return new LocationType();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.w3.org/ns/locn#", name = "poBox")
@Nonnull
public JAXBElement createPoBox(
@Nullable
final String value) {
return new JAXBElement(_PoBox_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.w3.org/ns/locn#", name = "thoroughfare")
@Nonnull
public JAXBElement createThoroughfare(
@Nullable
final String value) {
return new JAXBElement(_Thoroughfare_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.w3.org/ns/locn#", name = "locatorDesignator")
@Nonnull
public JAXBElement createLocatorDesignator(
@Nullable
final String value) {
return new JAXBElement(_LocatorDesignator_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.w3.org/ns/locn#", name = "locatorName")
@Nonnull
public JAXBElement createLocatorName(
@Nullable
final String value) {
return new JAXBElement(_LocatorName_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.w3.org/ns/locn#", name = "addressArea")
@Nonnull
public JAXBElement createAddressArea(
@Nullable
final String value) {
return new JAXBElement(_AddressArea_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.w3.org/ns/locn#", name = "postName")
@Nonnull
public JAXBElement createPostName(
@Nullable
final String value) {
return new JAXBElement(_PostName_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.w3.org/ns/locn#", name = "adminUnitLevel1")
@Nonnull
public JAXBElement createAdminUnitLevel1(
@Nullable
final String value) {
return new JAXBElement(_AdminUnitLevel1_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.w3.org/ns/locn#", name = "adminUnitLevel2")
@Nonnull
public JAXBElement createAdminUnitLevel2(
@Nullable
final String value) {
return new JAXBElement(_AdminUnitLevel2_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.w3.org/ns/locn#", name = "postCode")
@Nonnull
public JAXBElement createPostCode(
@Nullable
final String value) {
return new JAXBElement(_PostCode_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.w3.org/ns/locn#", name = "addressId")
@Nonnull
public JAXBElement createAddressId(
@Nullable
final String value) {
return new JAXBElement(_AddressId_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AddressType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link AddressType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.w3.org/ns/locn#", name = "address")
@Nonnull
public JAXBElement createAddress(
@Nullable
final AddressType value) {
return new JAXBElement(_Address_QNAME, AddressType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link GeometryType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link GeometryType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.w3.org/ns/locn#", name = "Geometry")
@Nonnull
public JAXBElement createGeometry(
@Nullable
final GeometryType value) {
return new JAXBElement(_Geometry_QNAME, GeometryType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocationType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocationType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.w3.org/ns/locn#", name = "Location")
@Nonnull
public JAXBElement createLocation(
@Nullable
final LocationType value) {
return new JAXBElement(_Location_QNAME, LocationType.class, null, value);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy