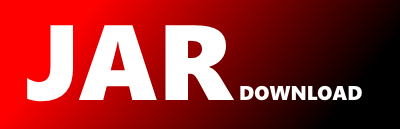
eu.toop.edm.jaxb.w3.odrl.Constraint Maven / Gradle / Ivy
package eu.toop.edm.jaxb.w3.odrl;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.annotation.Nonnegative;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAnyAttribute;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlID;
import javax.xml.bind.annotation.XmlIDREF;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.CollapsedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import javax.xml.namespace.QName;
import com.helger.commons.annotation.CodingStyleguideUnaware;
import com.helger.commons.annotation.ReturnsMutableCopy;
import com.helger.commons.annotation.ReturnsMutableObject;
import com.helger.commons.equals.EqualsHelper;
import com.helger.commons.hashcode.HashCodeGenerator;
import com.helger.commons.lang.IExplicitlyCloneable;
import com.helger.commons.string.ToStringGenerator;
/**
* Java class for Constraint complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="Constraint">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <attGroup ref="{http://www.w3.org/ns/odrl/2/}idAttributes"/>
* <attribute name="name" type="{http://www.w3.org/ns/odrl/2/}URIQnameQcode" />
* <attribute name="operator" type="{http://www.w3.org/ns/odrl/2/}URIQnameQcode" />
* <attribute name="rightOperand" type="{http://www.w3.org/ns/odrl/2/}listOfValues" />
* <attribute name="dataType" type="{http://www.w3.org/ns/odrl/2/}URIQnameQcode" />
* <attribute name="unit" type="{http://www.w3.org/ns/odrl/2/}URIQnameQcode" />
* <attribute name="status" type="{http://www.w3.org/2001/XMLSchema}string" />
* <anyAttribute processContents='lax' namespace='##other'/>
* </restriction>
* </complexContent>
* </complexType>
*
* This class was annotated by ph-jaxb22-plugin -Xph-annotate
* This class contains methods created by ph-jaxb22-plugin -Xph-equalshashcode
* This class contains methods created by ph-jaxb22-plugin -Xph-tostring
* This class contains methods created by ph-jaxb22-plugin -Xph-list-extension
* This class contains methods created by ph-jaxb22-plugin -Xph-cloneable2
* This class contains methods created by ph-jaxb22-plugin -Xph-value-extender
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Constraint")
@CodingStyleguideUnaware
public class Constraint implements Serializable, IExplicitlyCloneable
{
@XmlAttribute(name = "name")
private String name;
@XmlAttribute(name = "operator")
private String operator;
@XmlAttribute(name = "rightOperand")
private List rightOperand;
@XmlAttribute(name = "dataType")
private String dataType;
@XmlAttribute(name = "unit")
private String unit;
@XmlAttribute(name = "status")
private String status;
@XmlAttribute(name = "id")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlID
@XmlSchemaType(name = "ID")
private String id;
@XmlAttribute(name = "idref")
@XmlIDREF
@XmlSchemaType(name = "IDREF")
private Object idref;
@XmlAnyAttribute
private Map otherAttributes = new HashMap();
/**
* Default constructor
* Note: automatically created by ph-jaxb22-plugin -Xph-value-extender
*
*/
public Constraint() {
}
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link String }
*
*/
@Nullable
public String getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setName(
@Nullable
String value) {
this.name = value;
}
/**
* Gets the value of the operator property.
*
* @return
* possible object is
* {@link String }
*
*/
@Nullable
public String getOperator() {
return operator;
}
/**
* Sets the value of the operator property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOperator(
@Nullable
String value) {
this.operator = value;
}
/**
* Gets the value of the rightOperand property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the rightOperand property.
*
*
* For example, to add a new item, do as follows:
*
* getRightOperand().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
@Nonnull
@ReturnsMutableObject("JAXB implementation style")
public List getRightOperand() {
if (rightOperand == null) {
rightOperand = new ArrayList();
}
return this.rightOperand;
}
/**
* Gets the value of the dataType property.
*
* @return
* possible object is
* {@link String }
*
*/
@Nullable
public String getDataType() {
return dataType;
}
/**
* Sets the value of the dataType property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDataType(
@Nullable
String value) {
this.dataType = value;
}
/**
* Gets the value of the unit property.
*
* @return
* possible object is
* {@link String }
*
*/
@Nullable
public String getUnit() {
return unit;
}
/**
* Sets the value of the unit property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setUnit(
@Nullable
String value) {
this.unit = value;
}
/**
* Gets the value of the status property.
*
* @return
* possible object is
* {@link String }
*
*/
@Nullable
public String getStatus() {
return status;
}
/**
* Sets the value of the status property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setStatus(
@Nullable
String value) {
this.status = value;
}
/**
* Gets the value of the id property.
*
* @return
* possible object is
* {@link String }
*
*/
@Nullable
public String getId() {
return id;
}
/**
* Sets the value of the id property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setId(
@Nullable
String value) {
this.id = value;
}
/**
* Gets the value of the idref property.
*
* @return
* possible object is
* {@link Object }
*
*/
@Nullable
public Object getIdref() {
return idref;
}
/**
* Sets the value of the idref property.
*
* @param value
* allowed object is
* {@link Object }
*
*/
public void setIdref(
@Nullable
Object value) {
this.idref = value;
}
/**
* Gets a map that contains attributes that aren't bound to any typed property on this class.
*
*
* the map is keyed by the name of the attribute and
* the value is the string value of the attribute.
*
* the map returned by this method is live, and you can add new attribute
* by updating the map directly. Because of this design, there's no setter.
*
*
* @return
* always non-null
*/
@Nullable
public Map getOtherAttributes() {
return otherAttributes;
}
/**
* Created by ph-jaxb22-plugin -Xph-equalshashcode
*
*/
@Override
public boolean equals(final Object o) {
if (o == this) {
return true;
}
if ((o == null)||(!getClass().equals(o.getClass()))) {
return false;
}
final Constraint rhs = ((Constraint) o);
if (!EqualsHelper.equals(dataType, rhs.dataType)) {
return false;
}
if (!EqualsHelper.equals(id, rhs.id)) {
return false;
}
if (!EqualsHelper.equals(idref, rhs.idref)) {
return false;
}
if (!EqualsHelper.equals(name, rhs.name)) {
return false;
}
if (!EqualsHelper.equals(operator, rhs.operator)) {
return false;
}
if (!EqualsHelper.equals(otherAttributes, rhs.otherAttributes)) {
return false;
}
if (!EqualsHelper.equalsCollection(rightOperand, rhs.rightOperand)) {
return false;
}
if (!EqualsHelper.equals(status, rhs.status)) {
return false;
}
if (!EqualsHelper.equals(unit, rhs.unit)) {
return false;
}
return true;
}
/**
* Created by ph-jaxb22-plugin -Xph-equalshashcode
*
*/
@Override
public int hashCode() {
return new HashCodeGenerator(this).append(dataType).append(id).append(idref).append(name).append(operator).append(otherAttributes).append(rightOperand).append(status).append(unit).getHashCode();
}
/**
* Created by ph-jaxb22-plugin -Xph-tostring
*
*/
@Override
public String toString() {
return new ToStringGenerator(this).append("dataType", dataType).append("id", id).append("idref", idref).append("name", name).append("operator", operator).append("otherAttributes", otherAttributes).append("rightOperand", rightOperand).append("status", status).append("unit", unit).getToString();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param aList
* The new list member to set. May be null
.
*/
public void setRightOperand(
@Nullable
final List aList) {
rightOperand = aList;
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* true
if at least one item is contained, false
otherwise.
*/
public boolean hasRightOperandEntries() {
return (!getRightOperand().isEmpty());
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* true
if no item is contained, false
otherwise.
*/
public boolean hasNoRightOperandEntries() {
return getRightOperand().isEmpty();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* The number of contained elements. Always ≥ 0.
*/
@Nonnegative
public int getRightOperandCount() {
return getRightOperand().size();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param index
* The index to retrieve
* @return
* The element at the specified index. May be null
* @throws IndexOutOfBoundsException
* if the index is invalid!
*/
@Nullable
public String getRightOperandAtIndex(
@Nonnegative
final int index)
throws IndexOutOfBoundsException
{
return getRightOperand().get(index);
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param elem
* The element to be added. May not be null
.
*/
public void addRightOperand(
@Nonnull
final String elem) {
getRightOperand().add(elem);
}
/**
* This method clones all values from this
to the passed object. All data in the parameter object is overwritten!Created by ph-jaxb22-plugin -Xph-cloneable2
*
* @param ret
* The target object to clone to. May not be null
.
*/
public void cloneTo(
@Nonnull
Constraint ret) {
ret.dataType = dataType;
ret.id = id;
ret.idref = idref;
ret.name = name;
ret.operator = operator;
if (otherAttributes == null) {
ret.otherAttributes = null;
} else {
ret.otherAttributes = new HashMap(otherAttributes);
}
if (rightOperand == null) {
ret.rightOperand = null;
} else {
List retRightOperand = new ArrayList();
for (String aItem: getRightOperand()) {
retRightOperand.add(aItem);
}
ret.rightOperand = retRightOperand;
}
ret.status = status;
ret.unit = unit;
}
/**
* Created by ph-jaxb22-plugin -Xph-cloneable2
*
* @return
* The cloned object. Never null
.
*/
@Nonnull
@ReturnsMutableCopy
@Override
public Constraint clone() {
Constraint ret = new Constraint();
cloneTo(ret);
return ret;
}
}