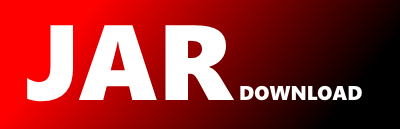
eu.trentorise.opendata.semantics.IntegrityChecker Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openentity-api Show documentation
Show all versions of openentity-api Show documentation
Api to access semantic services of open data in trentino project
The newest version!
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy