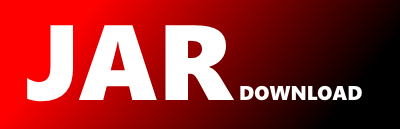
eu.webtoolkit.jwt.WPushButton Maven / Gradle / Ivy
Show all versions of jwt Show documentation
/*
* Copyright (C) 2009 Emweb bvba, Leuven, Belgium.
*
* See the LICENSE file for terms of use.
*/
package eu.webtoolkit.jwt;
import java.util.*;
import java.util.regex.*;
import java.io.*;
import java.lang.ref.*;
import java.util.concurrent.locks.ReentrantLock;
import javax.servlet.http.*;
import javax.servlet.*;
import eu.webtoolkit.jwt.*;
import eu.webtoolkit.jwt.chart.*;
import eu.webtoolkit.jwt.utils.*;
import eu.webtoolkit.jwt.servlet.*;
/**
* A widget that represents a push button.
*
*
* To act on a button click, connect a slot to the
* {@link WInteractWidget#clicked() WInteractWidget#clicked()} signal.
*
* WPushButton is an {@link WWidget#setInline(boolean inlined) inline} widget.
*
*
CSS
*
* The widget corresponds to the HTML <button>
tag and has
* the Wt-btn
style. It may be styled through the current theme, or
* you can override the style using internal or external CSS as appropriate.
*/
public class WPushButton extends WFormWidget {
/**
* Creates a push button.
*/
public WPushButton(WContainerWidget parent) {
super(parent);
this.text_ = new WString();
this.icon_ = "";
this.link_ = new WLink();
this.linkTarget_ = AnchorTarget.TargetSelf;
this.flags_ = new BitSet();
this.redirectJS_ = null;
}
/**
* Creates a push button.
*
* Calls {@link #WPushButton(WContainerWidget parent)
* this((WContainerWidget)null)}
*/
public WPushButton() {
this((WContainerWidget) null);
}
/**
* Creates a push button with given label text.
*/
public WPushButton(CharSequence text, WContainerWidget parent) {
super(parent);
this.text_ = WString.toWString(text);
this.icon_ = "";
this.link_ = new WLink();
this.linkTarget_ = AnchorTarget.TargetSelf;
this.flags_ = new BitSet();
this.redirectJS_ = null;
}
/**
* Creates a push button with given label text.
*
* Calls {@link #WPushButton(CharSequence text, WContainerWidget parent)
* this(text, (WContainerWidget)null)}
*/
public WPushButton(CharSequence text) {
this(text, (WContainerWidget) null);
}
public void remove() {
;
super.remove();
}
/**
* Sets the button text.
*/
public void setText(CharSequence text) {
if (canOptimizeUpdates() && text.equals(this.text_)) {
return;
}
this.text_ = WString.toWString(text);
this.flags_.set(BIT_TEXT_CHANGED);
this.repaint(EnumSet.of(RepaintFlag.RepaintInnerHtml));
}
/**
* Returns the button text.
*
*
* @see WPushButton#setText(CharSequence text)
*/
public WString getText() {
return this.text_;
}
/**
* Sets an icon.
*
* The icon is placed to the left of the text.
*/
public void setIcon(String url) {
if (canOptimizeUpdates() && url.equals(this.icon_)) {
return;
}
this.icon_ = url;
this.flags_.set(BIT_ICON_CHANGED);
this.repaint(EnumSet.of(RepaintFlag.RepaintInnerHtml));
}
/**
* Returns the icon.
*
*
* @see WPushButton#setIcon(String url)
*/
public String getIcon() {
return this.icon_;
}
/**
* Sets a destination link.
*
* This method can be used to make the button behave like a {@link WAnchor}
* (or conversely, an anchor look like a button) and redirect to another URL
* when clicked.
*
* The link
may be to a URL, a resource, or an internal path.
*
* By default, a button does not link to an URL and you should listen to the
* {@link WInteractWidget#clicked() WInteractWidget#clicked()} signal to
* react to a click event.
*/
public void setLink(WLink link) {
if (link.equals(this.link_)) {
return;
}
this.link_ = link;
this.flags_.set(BIT_LINK_CHANGED);
if (link.getType() == WLink.Type.Resource) {
link.getResource().dataChanged().addListener(this,
new Signal.Listener() {
public void trigger() {
WPushButton.this.resourceChanged();
}
});
}
this.repaint(EnumSet.of(RepaintFlag.RepaintPropertyIEMobile));
}
/**
* Returns the destination link.
*
*
* @see WPushButton#setLink(WLink link)
*/
public WLink getLink() {
return this.link_;
}
/**
* Sets a destination URL (deprecated).
*
*
* @deprecated Use {@link WPushButton#setLink(WLink link) setLink()}
* insteadd.
*/
public void setRef(String url) {
this.setLink(new WLink(url));
}
/**
* Returns the destination URL (deprecated).
*
* When the button refers to a resource, the current resource URL is
* returned. Otherwise, the URL is returned that was set using
* {@link WPushButton#setRef(String url) setRef()}.
*
*
* @see WPushButton#setRef(String url)
* @see WResource#getUrl()
* @deprecated Use {@link WPushButton#getLink() getLink()} instead.
*/
public String getRef() {
return this.link_.getUrl();
}
/**
* Sets a destination resource (deprecated).
*
* This method can be used to make the button behave like a {@link WAnchor}
* (or conversely, an anchor look like a button) and redirect to another
* resource when clicked.
*
* A resource specifies application-dependent content, which may be
* generated by your application on demand.
*
* This sets the resource
as the destination of the button, and
* is an alternative to {@link WPushButton#setRef(String url) setRef()}. The
* resource may be cleared by passing resource
=
* null
.
*
* The button does not assume ownership of the resource.
*
*
* @see WPushButton#setRef(String url)
* @deprecated Use {@link WPushButton#setLink(WLink link) setLink()}
* instead.
*/
public void setResource(WResource resource) {
this.setLink(new WLink(resource));
}
/**
* Returns the destination resource (deprecated).
*
* Returns null
if no resource has been set.
*
*
* @see WPushButton#setResource(WResource resource)
* @deprecated Use {@link WPushButton#getLink() getLink()} instead.
*/
public WResource getResource() {
return this.link_.getResource();
}
/**
* Sets the link target.
*
* This sets the target where the linked contents should be displayed. The
* default target is TargetSelf.
*/
public void setLinkTarget(AnchorTarget target) {
this.linkTarget_ = target;
}
/**
* Returns the location where the linked content should be displayed.
*
*
* @see WPushButton#setLinkTarget(AnchorTarget target)
*/
public AnchorTarget getLinkTarget() {
return this.linkTarget_;
}
public void refresh() {
if (this.text_.refresh()) {
this.flags_.set(BIT_TEXT_CHANGED);
this.repaint(EnumSet.of(RepaintFlag.RepaintInnerHtml));
}
super.refresh();
}
private static final int BIT_TEXT_CHANGED = 0;
private static final int BIT_ICON_CHANGED = 1;
private static final int BIT_ICON_RENDERED = 2;
private static final int BIT_LINK_CHANGED = 3;
private WString text_;
private String icon_;
private WLink link_;
private AnchorTarget linkTarget_;
BitSet flags_;
private JSlot redirectJS_;
void updateDom(DomElement element, boolean all) {
if (all) {
element.setAttribute("type", "button");
element.setProperty(Property.PropertyClass, "Wt-btn");
}
if (this.flags_.get(BIT_ICON_CHANGED) || all
&& this.icon_.length() != 0) {
DomElement image = DomElement
.createNew(DomElementType.DomElement_IMG);
image.setProperty(Property.PropertySrc, this.icon_);
image.setId("im" + this.getFormName());
element.insertChildAt(image, 0);
this.flags_.set(BIT_ICON_RENDERED);
}
if (this.flags_.get(BIT_TEXT_CHANGED) || all) {
element.setProperty(Property.PropertyInnerHTML, this.text_
.isLiteral() ? escapeText(this.text_, true).toString()
: this.text_.toString());
this.flags_.clear(BIT_TEXT_CHANGED);
}
if (this.flags_.get(BIT_LINK_CHANGED) || all && !this.link_.isNull()) {
if (!this.link_.isNull()) {
WApplication app = WApplication.getInstance();
if (!(this.redirectJS_ != null)) {
this.redirectJS_ = new JSlot();
this.clicked().addListener(this.redirectJS_);
if (!app.getEnvironment().hasAjax()) {
this.clicked().addListener(this,
new Signal1.Listener() {
public void trigger(WMouseEvent e1) {
WPushButton.this.doRedirect();
}
});
}
}
if (this.link_.getType() == WLink.Type.InternalPath) {
this.redirectJS_
.setJavaScript("function(){Wt3_1_11.history.navigate("
+ jsStringLiteral(this.link_
.getInternalPath()) + ",true);}");
} else {
if (this.linkTarget_ == AnchorTarget.TargetNewWindow) {
this.redirectJS_
.setJavaScript("function(){window.open("
+ jsStringLiteral(this.link_.getUrl())
+ ");}");
} else {
this.redirectJS_
.setJavaScript("function(){window.location="
+ jsStringLiteral(this.link_.getUrl())
+ ";}");
}
}
this.clicked().senderRepaint();
} else {
;
this.redirectJS_ = null;
}
}
super.updateDom(element, all);
}
DomElementType getDomElementType() {
return DomElementType.DomElement_BUTTON;
}
void propagateRenderOk(boolean deep) {
this.flags_.clear();
super.propagateRenderOk(deep);
}
void getDomChanges(List result, WApplication app) {
if (this.flags_.get(BIT_ICON_CHANGED)
&& this.flags_.get(BIT_ICON_RENDERED)) {
DomElement image = DomElement.getForUpdate("im"
+ this.getFormName(), DomElementType.DomElement_IMG);
if (this.icon_.length() == 0) {
image.removeFromParent();
this.flags_.clear(BIT_ICON_RENDERED);
} else {
image.setProperty(Property.PropertySrc, this.icon_);
}
result.add(image);
this.flags_.clear(BIT_ICON_CHANGED);
}
super.getDomChanges(result, app);
}
private void doRedirect() {
WApplication app = WApplication.getInstance();
if (!app.getEnvironment().hasAjax()) {
if (this.link_.getType() == WLink.Type.InternalPath) {
app.setInternalPath(this.link_.getInternalPath(), true);
} else {
app.redirect(this.link_.getUrl());
}
}
}
private void resourceChanged() {
this.flags_.set(BIT_LINK_CHANGED);
this.repaint(EnumSet.of(RepaintFlag.RepaintPropertyIEMobile));
}
}