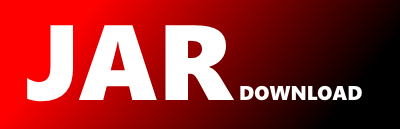
eu.webtoolkit.jwt.WXmlLocalizedStrings Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jwt Show documentation
Show all versions of jwt Show documentation
Java library for developing web applications
/*
* Copyright (C) 2009 Emweb bvba, Leuven, Belgium.
*
* See the LICENSE file for terms of use.
*/
package eu.webtoolkit.jwt;
import java.io.File;
import java.io.StringWriter;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import javax.xml.transform.Transformer;
import javax.xml.transform.TransformerConfigurationException;
import javax.xml.transform.TransformerException;
import javax.xml.transform.TransformerFactory;
import javax.xml.transform.dom.DOMSource;
import javax.xml.transform.stream.StreamResult;
import org.w3c.dom.Document;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
/**
* WXmlLocalizedStrings is a {@link WLocalizedStrings} implementation which uses an XML file as input resource.
*/
public class WXmlLocalizedStrings extends WLocalizedStrings {
private List bundleNames = new ArrayList();
private Map keyValues = new HashMap();
/**
* Constructor.
*/
public WXmlLocalizedStrings() {
}
/**
* Use an XML file as input resource.
*
* @param bundleName
*/
public void use(String bundleName) {
this.bundleNames.add(bundleName);
readXmlResource(bundleName);
}
public void refresh() {
keyValues.clear();
for (String bundleName : bundleNames)
readXmlResource(bundleName);
}
private void readXmlResource(String bundleName) {
WApplication app = WApplication.getInstance();
URL url = null;
for (String path : StringUtils.expandLocales(bundleName, WApplication.getInstance().getLocale().toString())) {
url = app.getClass().getResource(path + ".xml");
try {
if(url == null)
url = new URL(path);
else
break;
} catch (MalformedURLException e) {
}
}
if (url == null)
throw new RuntimeException("JWt exception: Could not find resource \"" + bundleName + "\"");
TransformerFactory tf = TransformerFactory.newInstance();
Transformer t = null;
try {
t = tf.newTransformer();
} catch (TransformerConfigurationException e1) {
throw new RuntimeException(e1);
}
DocumentBuilder docBuilder = null;
Document doc = null;
DocumentBuilderFactory docBuilderFactory = DocumentBuilderFactory.newInstance();
docBuilderFactory.setIgnoringElementContentWhitespace(true);
try {
docBuilder = docBuilderFactory.newDocumentBuilder();
} catch (ParserConfigurationException e) {
e.printStackTrace();
}
try {
doc = docBuilder.parse(url.openStream());
} catch (Exception e) {
e.printStackTrace();
}
NodeList nl = doc.getElementsByTagName("message");
if (nl.getLength() > 0) {
Node node;
for (int i = 0; i < nl.getLength(); i++) {
node = nl.item(i);
if (node.getNodeName().equals("message")) {
String id = node.getAttributes().getNamedItem("id").getNodeValue();
StringWriter writer = new StringWriter();
try {
t.transform(new DOMSource(node), new StreamResult(writer));
} catch (TransformerException e) {
throw new RuntimeException(e);
}
// Remove leading ...
String xml = writer.toString().substring(53 + id.length());
// ... and trailing
xml = xml.substring(0, xml.length() - 10);
keyValues.put(id, xml);
}
}
}
}
public String resolveKey(String key) {
if (keyValues != null && keyValues.get(key) != null)
return keyValues.get(key);
else
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy