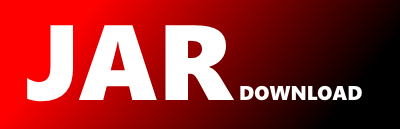
com.github.dynamicextensionsalfresco.osgi.BundleDependencies Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of alfresco-integration Show documentation
Show all versions of alfresco-integration Show documentation
Adds an OSGi container to alfresco repository supporting dynamic code reloading, classpath isolation and a bunch of other useful features
package com.github.dynamicextensionsalfresco.osgi;
import com.springsource.util.osgi.manifest.BundleManifest;
import com.springsource.util.osgi.manifest.BundleManifestFactory;
import com.springsource.util.osgi.manifest.ExportedPackage;
import com.springsource.util.osgi.manifest.ImportedPackage;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import org.jetbrains.annotations.NotNull;
import org.osgi.framework.Bundle;
/**
* Sort a list of bundles by their dependency graph. Bundles without or satisfied dependencies come first.
*
* @author Laurent Van der Linden.
* @author Toon Geens
*/
public final class BundleDependencies {
private static final BundleMetadataProvider bundleMetadataProvider = new BundleMetadataProvider();
private BundleDependencies() {
}
public static List sortByDependencies(List bundles) {
if (bundles.size() == 1) {
return bundles;
}
List descriptors = Mapper.map(bundles, new MappingFunc() {
@Override
public BundleDescriptor map(Bundle item) {
return new BundleDescriptor(item);
}
});
Collection sorted = DependencySorter.sort(descriptors, bundleMetadataProvider);
return Mapper.map(sorted, new MappingFunc() {
@Override
public Bundle map(BundleDescriptor item) {
return item.getBundle();
}
});
}
public static class BundleMetadataProvider implements
DependencyMetadataProvider {
@Override
public boolean allowCircularReferences() {
// SLF4J has a circular reference
return true;
}
@Override
public Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy