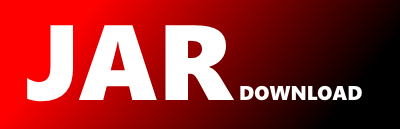
com.github.dynamicextensionsalfresco.osgi.spring.ServiceDefinitionConfigurationFactoryBean Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of alfresco-integration Show documentation
Show all versions of alfresco-integration Show documentation
Adds an OSGi container to alfresco repository supporting dynamic code reloading, classpath isolation and a bunch of other useful features
package com.github.dynamicextensionsalfresco.osgi.spring;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.LineNumberReader;
import java.util.ArrayList;
import java.util.List;
import com.github.dynamicextensionsalfresco.osgi.ServiceDefinition;
import com.github.dynamicextensionsalfresco.osgi.ServiceDefinitionEditor;
import org.apache.commons.collections.list.AbstractLinkedList;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.FactoryBean;
import org.springframework.core.io.Resource;
/**
* {@link FactoryBean} for creating a List of {@link ServiceDefinition}s from a text file.
*
* @author Laurens Fridael
*
*/
public class ServiceDefinitionConfigurationFactoryBean extends
AbstractConfigurationFileFactoryBean> {
private final Logger logger = LoggerFactory.getLogger(getClass());
/* State */
private List serviceDefinitions;
/* Operations */
@SuppressWarnings("unchecked")
@Override
public Class extends List> getObjectType() {
return (Class extends List>) (Class>) List.class;
}
@Override
public boolean isSingleton() {
return true;
}
@Override
public List getObject() throws IOException {
if (serviceDefinitions == null) {
serviceDefinitions = createServiceDefinitions();
}
return serviceDefinitions;
}
/* Utility operations */
protected List createServiceDefinitions() throws IOException {
final List serviceDefinitions = new ArrayList();
final ServiceDefinitionEditor serviceDefinitionEditor = new ServiceDefinitionEditor();
for (final Resource configuration : resolveConfigurations()) {
final LineNumberReader in = new LineNumberReader(new InputStreamReader(configuration.getInputStream()));
for (String line; (line = in.readLine()) != null;) {
line = line.trim();
if (line.isEmpty() || line.startsWith("#")) {
// Skip empty lines and comments.
continue;
}
try {
serviceDefinitionEditor.setAsText(line);
final ServiceDefinition serviceDefinition = (ServiceDefinition) serviceDefinitionEditor.getValue();
serviceDefinitions.add(serviceDefinition);
} catch (final IllegalArgumentException e) {
logger.warn("Could not parse SystemPackage configuration line: {}", e.getMessage());
}
}
}
return serviceDefinitions;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy