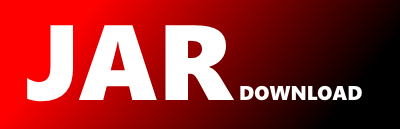
com.github.dynamicextensionsalfresco.webscripts.HandlerMethods Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of annotations-runtime Show documentation
Show all versions of annotations-runtime Show documentation
Adds an OSGi container to alfresco repository supporting dynamic code reloading, classpath isolation and a bunch of other useful features
package com.github.dynamicextensionsalfresco.webscripts;
import com.github.dynamicextensionsalfresco.webscripts.annotations.Attribute;
import com.github.dynamicextensionsalfresco.webscripts.annotations.Before;
import com.github.dynamicextensionsalfresco.webscripts.annotations.ResponseTemplate;
import com.github.dynamicextensionsalfresco.webscripts.annotations.Uri;
import org.springframework.core.annotation.AnnotationUtils;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.List;
/**
* Parameter object for specifying {@link Uri}, {@link Before} and {@link Attribute}-annotated Web Script handler
* methods.
*
* @author Laurens Fridael
*
*/
public class HandlerMethods {
private final List beforeMethods = new ArrayList();
private final List attributeMethods = new ArrayList();
private Method uriMethod;
private final List exceptionHandlerMethods = new ArrayList();
public List getBeforeMethods() {
return beforeMethods;
}
public List getAttributeMethods() {
return attributeMethods;
}
public Method getUriMethod() {
return uriMethod;
}
public List getExceptionHandlerMethods() {
return exceptionHandlerMethods;
}
public List findExceptionHandlers(final Throwable exception) {
final List handlerMethods = new ArrayList(1);
for (final ExceptionHandlerMethod exceptionHandlerMethod : getExceptionHandlerMethods()) {
if (exceptionHandlerMethod.canHandle(exception)) {
handlerMethods.add(exceptionHandlerMethod.getMethod());
}
}
return handlerMethods;
}
public boolean useResponseTemplate() {
return (AnnotationUtils.findAnnotation(uriMethod, ResponseTemplate.class) != null);
}
public String getResponseTemplateName() {
final ResponseTemplate responseTemplate = AnnotationUtils.findAnnotation(uriMethod, ResponseTemplate.class);
return (responseTemplate != null ? responseTemplate.value() : null);
}
/**
* Creates a new instance for the specified {@link Uri}-annotated method.
*
* @param uriMethod
*/
public HandlerMethods createForUriMethod(final Method uriMethod) {
final HandlerMethods handlerMethods = new HandlerMethods();
handlerMethods.beforeMethods.addAll(getBeforeMethods());
handlerMethods.attributeMethods.addAll(getAttributeMethods());
handlerMethods.uriMethod = uriMethod;
handlerMethods.exceptionHandlerMethods.addAll(getExceptionHandlerMethods());
return handlerMethods;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy