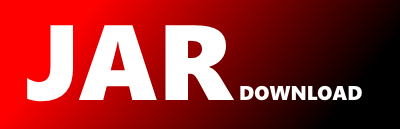
com.github.dynamicextensionsalfresco.actions.DefaultActionExecuterRegistry Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of annotations-runtime Show documentation
Show all versions of annotations-runtime Show documentation
Adds an OSGi container to alfresco repository supporting dynamic code reloading, classpath isolation and a bunch of other useful features
package com.github.dynamicextensionsalfresco.actions;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import org.alfresco.repo.action.executer.ActionExecuter;
import org.springframework.context.ApplicationContext;
import org.springframework.context.ApplicationContextAware;
public class DefaultActionExecuterRegistry implements ActionExecuterRegistry, ApplicationContextAware {
/* Dependencies */
private ApplicationContext applicationContext;
/* State */
private final Map actionExecutersByName = new ConcurrentHashMap();
/* Operations */
@Override
public boolean hasActionExecuter(final String name) {
/* Note: we must verify the ApplicationContext for matching bean names as well. */
return actionExecutersByName.containsKey(name) || applicationContext.containsBeanDefinition(name);
}
@Override
public ActionExecuter getActionExecuter(final String name) {
return actionExecutersByName.get(name);
}
@Override
public void registerActionExecuter(final ActionExecuter actionExecuter) {
final String name = actionExecuter.getActionDefinition().getName();
if (hasActionExecuter(name)) {
throw new IllegalStateException("Duplicate ActionExecuter " + name);
}
getActionExecutersByName().put(name, actionExecuter);
}
@Override
public void unregisterActionExecuter(final ActionExecuter actionExecuter) {
if (getActionExecutersByName().containsValue(actionExecuter)) {
actionExecutersByName.remove(actionExecuter.getActionDefinition().getName());
}
}
/* Dependencies */
@Override
public void setApplicationContext(final ApplicationContext applicationContext) {
this.applicationContext = applicationContext;
}
/* State */
protected Map getActionExecutersByName() {
return actionExecutersByName;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy