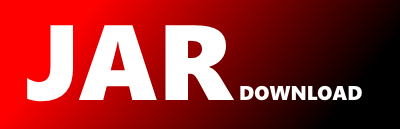
com.github.dynamicextensionsalfresco.quartz.QuartzJobRegistrar Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of annotations-runtime Show documentation
Show all versions of annotations-runtime Show documentation
Adds an OSGi container to alfresco repository supporting dynamic code reloading, classpath isolation and a bunch of other useful features
package com.github.dynamicextensionsalfresco.quartz;
import com.github.dynamicextensionsalfresco.jobs.ScheduledQuartzJob;
import org.alfresco.schedule.AbstractScheduledLockedJob;
import org.quartz.*;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.DisposableBean;
import org.springframework.beans.factory.InitializingBean;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.ApplicationContext;
import org.springframework.context.ApplicationContextAware;
import org.springframework.util.Assert;
import java.text.ParseException;
import java.util.ArrayList;
import java.util.Map;
import java.util.Properties;
/**
* Created by jasper on 19/07/17.
*/
public class QuartzJobRegistrar implements ApplicationContextAware, InitializingBean, DisposableBean {
private Logger logger = LoggerFactory.getLogger(QuartzJobRegistrar.class);
public final static String BEAN_ID="bean";
@Autowired
protected Scheduler scheduler;
private ArrayList registeredJobs = new ArrayList();
private ApplicationContext applicationContext;
@Override
public void afterPropertiesSet() throws ParseException, SchedulerException {
Map scheduledBeans = applicationContext.getBeansWithAnnotation(ScheduledQuartzJob.class);
for (Map.Entry entry : scheduledBeans.entrySet()) {
Object bean = entry.getValue();
Assert.isInstanceOf(Job.class, bean, "annotated Quartz job classes should implement org.quartz.Job");
ScheduledQuartzJob annotation = bean.getClass().getAnnotation(ScheduledQuartzJob.class);
try {
String cron = applicationContext.getBean("global-properties", Properties.class).getProperty(annotation.cronProp(), annotation.cron());
CronTrigger trigger = new CronTrigger(annotation.name(), annotation.group(), cron);
JobDetail jobDetail = new JobDetail(annotation.name(), annotation.group(), annotation.cluster() ? AbstractScheduledLockedJob.class : GenericQuartzJob.class);
JobDataMap map = new JobDataMap();
map.put(BEAN_ID, bean);
jobDetail.setJobDataMap(map);
scheduler.scheduleJob(jobDetail, trigger);
registeredJobs.add(annotation);
logger.debug("scheduled job " + annotation.name() + " from group " + annotation.group() + " using cron " + annotation.cron());
} catch (Exception e) {
logger.error("failed to register job " + annotation.name() + " using cron " + annotation.group(), e);
}
}
}
@Override
public void destroy() throws SchedulerException {
for (ScheduledQuartzJob job : registeredJobs) {
try {
scheduler.unscheduleJob(job.name(), job.group());
logger.debug("unscheduled job " + job.name() + " from group " + job.group());
} catch (SchedulerException e) {
logger.error("failed to cleanup quartz job " + job, e);
}
}
}
public void setApplicationContext(ApplicationContext applicationContext) {
this.applicationContext = applicationContext;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy