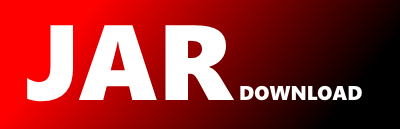
com.github.dynamicextensionsalfresco.webscripts.arguments.RequestBodyArgumentResolver Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of annotations-runtime Show documentation
Show all versions of annotations-runtime Show documentation
Adds an OSGi container to alfresco repository supporting dynamic code reloading, classpath isolation and a bunch of other useful features
package com.github.dynamicextensionsalfresco.webscripts.arguments;
import com.github.dynamicextensionsalfresco.webscripts.MessageConverterRegistry;
import com.github.dynamicextensionsalfresco.webscripts.messages.AnnotationWebScriptInputMessage;
import org.springframework.extensions.webscripts.WebScriptRequest;
import org.springframework.extensions.webscripts.WebScriptResponse;
import org.springframework.http.MediaType;
import org.springframework.http.converter.HttpMessageConverter;
import org.springframework.web.bind.annotation.RequestBody;
import java.io.IOException;
import java.io.InputStream;
import java.lang.annotation.Annotation;
public class RequestBodyArgumentResolver implements ArgumentResolver
© 2015 - 2025 Weber Informatics LLC | Privacy Policy