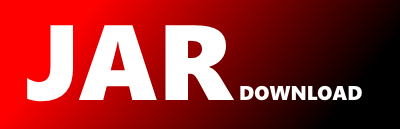
com.github.dynamicextensionsalfresco.blueprint.spring3.Spring3OsgiAutowireBeanFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of blueprint-integration-spring-3 Show documentation
Show all versions of blueprint-integration-spring-3 Show documentation
Adds an OSGi container to alfresco repository supporting dynamic code reloading, classpath isolation and a bunch of other useful features
package com.github.dynamicextensionsalfresco.blueprint.spring3;
import com.github.dynamicextensionsalfresco.BeanNames;
import com.github.dynamicextensionsalfresco.annotations.AlfrescoService;
import com.github.dynamicextensionsalfresco.annotations.ServiceType;
import java.lang.annotation.Annotation;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import org.osgi.framework.BundleContext;
import org.osgi.framework.ServiceReference;
import org.springframework.beans.factory.BeanFactory;
import org.springframework.beans.factory.config.DependencyDescriptor;
import org.springframework.beans.factory.support.DefaultListableBeanFactory;
import org.springframework.util.ClassUtils;
import org.springframework.util.StringUtils;
/**
* Spring 3 compatible {@link BeanFactory} that
*
* - Augments default autowiring logic by attempting to resolve dependencies using Alfresco naming conventions.
* - Resolves dependencies on OSGi services or the {@link BundleContext}
*
*
* @author Laurens Fridael
*/
public class Spring3OsgiAutowireBeanFactory extends DefaultListableBeanFactory {
private final BundleContext bundleContext;
private final Set internalBeanNames = new HashSet<>();
public Spring3OsgiAutowireBeanFactory(final BeanFactory parentBeanFactory, final BundleContext bundleContext) {
super(parentBeanFactory);
this.bundleContext = bundleContext;
for (BeanNames beanName : BeanNames.values()) {
internalBeanNames.add(beanName.id());
}
}
/* Main operations */
@Override
@SuppressWarnings({"rawtypes", "unchecked"})
protected Map findAutowireCandidates(final String beanName, final Class requiredType,
final DependencyDescriptor descriptor) {
Map candidateBeansByName = Collections.emptyMap();
if (BundleContext.class.isAssignableFrom(requiredType)) {
candidateBeansByName = new HashMap<>(1);
candidateBeansByName.put(requiredType.getName(), bundleContext);
} else {
final ServiceReference serviceReference = bundleContext.getServiceReference(requiredType);
if (serviceReference != null) {
candidateBeansByName = new HashMap<>(1);
candidateBeansByName.put(requiredType.getName(), bundleContext.getService(serviceReference));
}
}
if (candidateBeansByName.isEmpty()) {
candidateBeansByName = super.findAutowireCandidates(beanName, requiredType, descriptor);
}
return candidateBeansByName;
}
@Override
protected String determinePrimaryCandidate(final Map candidateBeans,
final DependencyDescriptor descriptor) {
String beanName = ClassUtils.getShortName(descriptor.getDependencyType());
for (String id : candidateBeans.keySet()) {
if (internalBeanNames.contains(id)) {
return id;
}
}
final AlfrescoService alfrescoService = getAnnotation(descriptor, AlfrescoService.class);
final ServiceType serviceType = alfrescoService != null ? alfrescoService.value() : ServiceType.DEFAULT;
switch (serviceType) {
default:
// Fall through
case DEFAULT:
if (candidateBeans.containsKey(beanName)) {
return beanName;
}
// Fall through
case LOW_LEVEL:
beanName = StringUtils.uncapitalize(beanName);
if (candidateBeans.containsKey(beanName)) {
return beanName;
}
break;
}
return super.determinePrimaryCandidate(candidateBeans, descriptor);
}
/* Utility operations */
@SuppressWarnings("unchecked")
private T getAnnotation(final DependencyDescriptor descriptor,
final Class annotationType) {
for (final Annotation annotation : descriptor.getAnnotations()) {
if (annotationType.isAssignableFrom(annotation.annotationType())) {
return (T) annotation;
}
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy