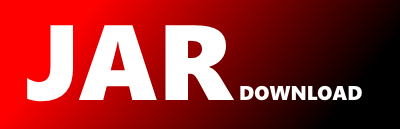
fi.evolver.basics.spring.common.ConfigurationRepository Maven / Gradle / Ivy
package fi.evolver.basics.spring.common;
import java.net.URI;
import java.net.URL;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import java.util.stream.Collectors;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.Pageable;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.data.jpa.repository.Query;
import org.springframework.stereotype.Repository;
import org.springframework.transaction.annotation.Propagation;
import org.springframework.transaction.annotation.Transactional;
import fi.evolver.basics.spring.common.entity.Configuration;
@Repository
@Transactional(propagation = Propagation.REQUIRED, readOnly = true)
public interface ConfigurationRepository extends JpaRepository {
static final Logger LOG = LoggerFactory.getLogger(ConfigurationRepository.class);
Configuration findFirstByKeyOrderByIdDesc(String key);
@Query("SELECT c FROM Configuration c WHERE " +
" c.active = true AND " +
" c.id = (SELECT max(c2.id) FROM Configuration c2 WHERE c2.key = c.key) " +
"ORDER BY" +
" c.key ASC")
Page findAllActive(Pageable page);
public default Optional getString(String key) {
return Optional.ofNullable(findFirstByKeyOrderByIdDesc(key))
.flatMap(c -> map(String.class, c, Object::toString));
}
public default List getList(String key) {
return getString(key)
.map(s -> s.split(","))
.map(Arrays::asList)
.orElse(Collections.emptyList()).stream()
.map(String::trim)
.filter(s -> !s.isEmpty())
.collect(Collectors.toList());
}
public default Optional getBoolean(String key) {
return Optional.ofNullable(findFirstByKeyOrderByIdDesc(key))
.flatMap(c -> map(Boolean.class, c, ConfigurationRepository::mapToBoolean));
}
private static Boolean mapToBoolean(String value) {
if ("true".equalsIgnoreCase(value))
return true;
if ("false".equalsIgnoreCase(value))
return false;
throw new IllegalArgumentException("invalid boolean");
}
public default Optional getInteger(String key) {
return Optional.ofNullable(findFirstByKeyOrderByIdDesc(key))
.flatMap(c -> map(Integer.class, c, Integer::parseInt));
}
public default Optional getLong(String key) {
return Optional.ofNullable(findFirstByKeyOrderByIdDesc(key))
.flatMap(c -> map(Long.class, c, Long::parseLong));
}
public default Optional getUri(String key) {
return Optional.ofNullable(findFirstByKeyOrderByIdDesc(key))
.flatMap(c -> map(URI.class, c, URI::new));
}
public default Optional getUrl(String key) {
return Optional.ofNullable(findFirstByKeyOrderByIdDesc(key))
.flatMap(c -> map(URL.class, c, URL::new));
}
public default Optional getLocalDate(String key) {
return Optional.ofNullable(findFirstByKeyOrderByIdDesc(key))
.flatMap(c -> map(LocalDate.class, c, LocalDate::parse));
}
public default Optional getLocalDateTime(String key) {
return Optional.ofNullable(findFirstByKeyOrderByIdDesc(key))
.flatMap(c -> map(LocalDateTime.class, c, LocalDateTime::parse));
}
private static Optional map(Class clazz, Configuration configuration, ValueMapper mapper) {
if (!configuration.isActive())
return Optional.empty();
try {
return Optional.ofNullable(mapper.apply(configuration.getValue()));
}
catch (Exception e) {
LOG.warn("CONFIGURATION value {} for key {} could not be mapped to {}: {}", configuration.getKey(), configuration.getValue(), clazz.getSimpleName(), e.getMessage());
return Optional.empty();
}
}
interface ValueMapper {
public T apply(String value) throws Exception;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy