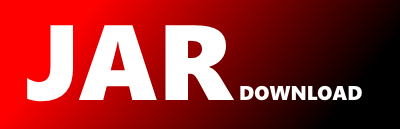
fi.evolver.basics.spring.common.entity.Configuration Maven / Gradle / Ivy
package fi.evolver.basics.spring.common.entity;
import java.io.Serializable;
import java.time.LocalDateTime;
import jakarta.persistence.*;
@Entity
@Table(name="configuration")
public class Configuration implements Serializable {
private static final long serialVersionUID = 1L;
@Id
@GeneratedValue(strategy=GenerationType.IDENTITY)
private long id;
@Column(name="key")
private String key;
@Column(name="value")
private String value;
@Column(name="username")
private String username;
@Column(name="created")
private LocalDateTime created;
@Column(name="active")
private boolean active;
@Column(name="comment")
private String comment;
public Configuration() { }
private Configuration(
String key,
String value,
String username,
LocalDateTime created,
boolean active,
String comment) {
this.key = key;
this.value = value;
this.username = username;
this.created = created;
this.active = active;
this.comment = comment;
}
public long getId() {
return this.id;
}
public String getKey() {
return this.key;
}
public String getValue() {
return this.value;
}
public String getUsername() {
return username;
}
public LocalDateTime getCreated() {
return created;
}
public boolean isActive() {
return active;
}
public String getComment() {
return comment;
}
public void setId(long id) {
this.id = id;
}
public Configuration makeInactive() {
return new Configuration(key, value, "?", LocalDateTime.now(), false, "Deleted");
}
@Override
public String toString() {
return String.format("%s: %s", key, value);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy