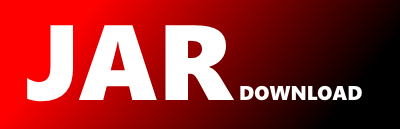
fi.evolver.basics.spring.common.entity.Sequence Maven / Gradle / Ivy
package fi.evolver.basics.spring.common.entity;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonIgnore;
import jakarta.persistence.*;
@Entity
@Table(name="application_seq_data")
public class Sequence implements Serializable {
private static final long serialVersionUID = 1L;
@Id
@GeneratedValue(strategy=GenerationType.IDENTITY)
private long id;
@Column(name="name")
private String name;
@Column(name="next_value")
private long nextValue;
public Sequence(String name, long nextValue) {
setName(name);
setNextValue(nextValue);
}
public Sequence() { }
public long getId() {
return this.id;
}
public String getName() {
return this.name;
}
public void setName(String name) {
this.name = name;
}
public long getNextValue() {
return this.nextValue;
}
public void setNextValue(long nextValue) {
this.nextValue = nextValue;
}
@Transient
@JsonIgnore
public long getAndIncrement() {
return nextValue++;
}
@Override
public String toString() {
return String.format("%s: %s", name, nextValue);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy