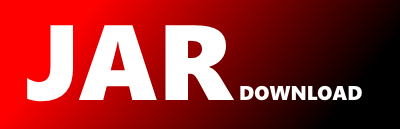
fi.evolver.basics.spring.http.HttpResponse Maven / Gradle / Ivy
package fi.evolver.basics.spring.http;
import java.io.Serializable;
import java.net.http.HttpTimeoutException;
import java.util.List;
import java.util.Map;
import org.springframework.http.HttpStatus;
public class HttpResponse implements Serializable {
private static final long serialVersionUID = 2525818118134201357L;
private final String statusCode;
private final String statusMessage;
private final String message;
private final Map> headers;
private final boolean success;
/**
* Creates a new HttpResponse.
*
* @param statusCode The HTTP response code or something informative if not available.
* @param statusMessage The HTTP response message or something informative if not available.
* @param message The actual (possibly error) response.
* @param headers Any headers returned by the server.
*/
public HttpResponse(String statusCode, String statusMessage, String message, Map> headers, boolean success) {
this.statusCode = statusCode;
this.statusMessage = statusMessage;
this.message = message;
this.headers = headers;
this.success = success;
}
/**
* The HTTP response code. Text in the case of an IO problem or timeout.
*
* @return HTTP response code or a textual message if we have problems below the HTTP level.
*/
public String getStatusCode() {
return statusCode;
}
/**
* HTTP response message. In the case of an IO problem or timeout, a description of the problem.
*
* @return HTTP response message or a description of the problem if we have problems below the HTTP level.
*/
public String getStatusMessage() {
return statusMessage;
}
/**
* The actual response document. In the case of an IO problem or timeout, null.
*
* @return HTTP response document or null if we have problems below the HTTP level.
*/
public String getMessage() {
return message;
}
/**
* HTTP response headers. In theory one header may have multiple values.
*
* @return HTTP response headers.
*/
public Map> getHeaders() {
return headers;
}
/**
* Get a specific HTTP header. If the header has multiple values, the first is returned.
* If the header has no values at all, returns null.
*
* @param headerName The name of the header to get.
* @return The first value of the specified header or null if it has no value or is not specified.
*/
public String getHeader(String headerName) {
List values = headers.get(headerName);
return values == null || values.isEmpty() ? null : values.get(0);
}
/**
* Whether the response and status code passed validations.
*
* @return True if the request was deemed successful.
*/
public boolean isSuccess() {
return success;
}
static HttpResponse create(java.net.http.HttpResponse httpResponse, boolean success) {
String statusCode = Integer.toString(httpResponse.statusCode());
String statusMessage = HttpStatus.valueOf(httpResponse.statusCode()).getReasonPhrase();
return new HttpResponse(
statusCode,
statusMessage,
httpResponse.body(),
httpResponse.headers().map(),
success);
}
static HttpResponse create(Throwable throwable) {
String statusCode = "ERROR";
if (throwable instanceof HttpTimeoutException)
statusCode = "TIMEOUT";
if (throwable instanceof InterruptedException)
statusCode = "INTERRUPTED";
return new HttpResponse(
statusCode,
throwable.toString(),
null,
Map.of(),
false);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy