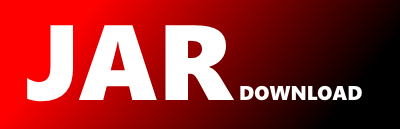
fi.evolver.basics.spring.lock.LockService Maven / Gradle / Ivy
package fi.evolver.basics.spring.lock;
import java.time.Duration;
import java.time.Instant;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import fi.evolver.basics.spring.lock.entity.Lock;
/**
* Provides database-persisted non-renewable locks that can be used for cluster-wide exclusive access.
*/
@Service
public class LockService {
private static final int RETRY_INTERVAL_MS = 1000;
private final LockRepository lockRepository;
@Autowired
private LockService(LockRepository lockRepository) {
this.lockRepository = lockRepository;
}
public LockHandle takeLock(String name, Duration maxValidity, Duration acquireRetryDuration) throws LockException {
Instant retryThreshold = Instant.now().plus(acquireRetryDuration).minusMillis(RETRY_INTERVAL_MS);
Lock lock;
while ((lock = lockRepository.tryTakeLock(name, maxValidity)) == null && Instant.now().isBefore(retryThreshold)) {
try {
Thread.sleep(RETRY_INTERVAL_MS);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new LockException(name);
}
}
if (lock == null)
throw new LockException(name);
return new LockHandle(lockRepository, lock);
}
/**
* @deprecated use {@link #takeLock(String, Duration, Duration)}
*/
@Deprecated
public LockHandle takeLock(String name, int validMs, int tryForMs) throws LockException {
return takeLock(name, Duration.ofMillis(validMs), Duration.ofMillis(tryForMs));
}
public LockHandle takeLock(String name, Duration maxValidity) throws LockException {
Lock lock = lockRepository.tryTakeLock(name, maxValidity);
if (lock == null)
throw new LockException(name);
return new LockHandle(lockRepository, lock);
}
/**
* @deprecated use {@link #takeLock(String, Duration)}
*/
@Deprecated
public LockHandle takeLock(String name, int validMs) throws LockException {
return takeLock(name, Duration.ofMillis(validMs));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy