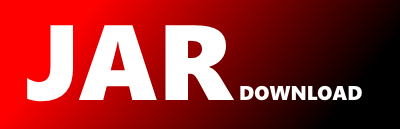
fi.evolver.basics.spring.timer.ScheduleController Maven / Gradle / Ivy
package fi.evolver.basics.spring.timer;
import java.time.Instant;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpStatus;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseStatus;
import org.springframework.web.bind.annotation.RestController;
import fi.evolver.basics.spring.http.MessageType;
import fi.evolver.basics.spring.http.crud.CrudController;
import fi.evolver.basics.spring.http.exception.HttpNotFoundException;
import fi.evolver.basics.spring.timer.entity.ScheduledTask;
import fi.evolver.basics.spring.timer.entity.ScheduledTask.State;
import io.swagger.v3.oas.annotations.Operation;
import io.swagger.v3.oas.annotations.media.Content;
import io.swagger.v3.oas.annotations.responses.ApiResponse;
import io.swagger.v3.oas.annotations.responses.ApiResponses;
@RestController
@RequestMapping("/schedule")
public class ScheduleController extends CrudController {
@Autowired
public ScheduleController(ScheduledTaskRepository scheduledTaskRepository) {
super(scheduledTaskRepository);
}
@Operation(summary = "Update scheduled task state")
@ApiResponses(value = {
@ApiResponse(responseCode = "204", description = "OK", content = @Content),
@ApiResponse(responseCode = "400", description = "Invalid request variables", content = @Content),
@ApiResponse(responseCode = "500", description = "Failed handling request", content = @Content)
})
@MessageType("ScheduledTask/update")
@ResponseStatus(value = HttpStatus.NO_CONTENT)
@PostMapping(value = "/{id}/state/{state}")
public void updateState(@PathVariable long id, @PathVariable State state) {
ScheduledTask task = jpaRepository.findById(id).orElseThrow(HttpNotFoundException::new);
task.setState(state);
jpaRepository.saveAndFlush(task);
}
@Operation(summary = "Set scheduled task to run on the next cycle")
@ApiResponses(value = {
@ApiResponse(responseCode = "204", description = "OK", content = @Content),
@ApiResponse(responseCode = "400", description = "Invalid request variables", content = @Content),
@ApiResponse(responseCode = "404", description = "Not found", content = @Content),
@ApiResponse(responseCode = "500", description = "Failed handling request", content = @Content)
})
@MessageType("ScheduledTask/run")
@ResponseStatus(value = HttpStatus.NO_CONTENT)
@PostMapping(value = "/{id}/run")
public void run(@PathVariable long id) {
ScheduledTask task = jpaRepository.findById(id).orElseThrow(HttpNotFoundException::new);
task.setNextFireTime(Instant.now().toEpochMilli());
jpaRepository.saveAndFlush(task);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy