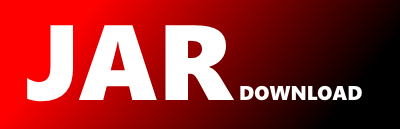
fi.evolver.basics.spring.messaging.SendResult Maven / Gradle / Ivy
package fi.evolver.basics.spring.messaging;
import java.util.Collections;
import java.util.Map;
import fi.evolver.utils.NullSafetyUtils;
import fi.evolver.utils.format.FormatUtils;
public class SendResult {
private final boolean success;
private final String responseBody;
private final Map responseDetails;
public SendResult(boolean success, String responseBody, Map responseDetails) {
this.success = success;
this.responseBody = responseBody;
this.responseDetails = NullSafetyUtils.denull(responseDetails, Collections.emptyMap());
}
public boolean isSuccess() {
return success;
}
public String getResponseBody() {
return responseBody;
}
public Map getResponseDetails() {
return responseDetails;
}
public static SendResult error(String errorMessage, Object... args) {
return new SendResult(false, null, Collections.singletonMap("ErrorMessage", FormatUtils.format(errorMessage, args)));
}
public static SendResult failure(String responseBody, Map responseDetails) {
return new SendResult(false, responseBody, responseDetails);
}
public static SendResult failure(Map responseDetails) {
return new SendResult(false, null, responseDetails);
}
public static SendResult success(String responseBody, Map responseDetails) {
return new SendResult(true, responseBody, responseDetails);
}
public static SendResult success(Map responseDetails) {
return new SendResult(true, null, responseDetails);
}
public static SendResult success() {
return new SendResult(true, null, Collections.emptyMap());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy