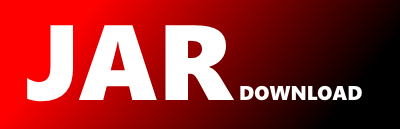
fi.evolver.utils.ContextUtils Maven / Gradle / Ivy
package fi.evolver.utils;
import java.util.*;
import java.util.function.Supplier;
import fi.evolver.utils.attribute.ContextAttribute;
import fi.evolver.utils.attribute.ContextRegistrableAttribute;
public class ContextUtils {
private static final ThreadLocal
© 2015 - 2025 Weber Informatics LLC | Privacy Policy