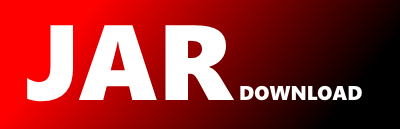
fi.evolver.utils.DateUtils Maven / Gradle / Ivy
package fi.evolver.utils;
import java.time.LocalDateTime;
import java.time.OffsetDateTime;
import java.time.ZoneId;
import java.time.ZonedDateTime;
import java.time.format.DateTimeFormatter;
public class DateUtils {
public static final int MS_IN_SECOND = 1000;
public static final int MS_IN_MINUTE = 60 * MS_IN_SECOND;
public static final int MS_IN_HOUR = 60 * MS_IN_MINUTE;
public static final int MS_IN_DAY = 24 * MS_IN_HOUR;
public static final ZoneId ZONE_HELSINKI = ZoneId.of("Europe/Helsinki");
public static final ZoneId ZONE_UTC = ZoneId.of("UTC");
public static final DateTimeFormatter FORMAT_DATE_FI = DateTimeFormatter.ofPattern("dd.MM.yyyy");
public static final DateTimeFormatter FORMAT_DATE_TIME_FI = DateTimeFormatter.ofPattern("dd.MM.yyyy HH:mm:ss");
private DateUtils() { }
/**
* Converts a LocalDateTime into an OffsetDateTime with the Europe/Helsinki zone's offset.
*
* @param timestamp The value to convert.
* @return The converted value.
*/
public static OffsetDateTime toOffsetDateTimeFi(LocalDateTime timestamp) {
return toOffsetDateTime(timestamp, ZONE_HELSINKI);
}
/**
* Converts a LocalDateTime into an OffsetDateTime with the given time zone's offset.
*
* @param timestamp The value to convert.
* @param zoneId The target time zone.
* @return The converted value.
*/
public static OffsetDateTime toOffsetDateTime(LocalDateTime timestamp, ZoneId zoneId) {
if (timestamp == null)
return null;
return timestamp.atZone(zoneId).toOffsetDateTime();
}
/**
* Converts a LocalDateTime into a ZonedDateTime at the Europe/Helsinki zone.
*
* @param timestamp The value to convert.
* @return The converted value.
*/
public static ZonedDateTime toZonedDateTimeFi(LocalDateTime timestamp) {
return toZonedDateTime(timestamp, ZONE_HELSINKI);
}
/**
* Converts a LocalDateTime into a ZonedDateTime at the given time zone.
*
* @param timestamp The value to convert.
* @param zoneId The target time zone.
* @return The converted value.
*/
public static ZonedDateTime toZonedDateTime(LocalDateTime timestamp, ZoneId zoneId) {
if (timestamp == null)
return null;
return timestamp.atZone(zoneId);
}
/**
* Converts an OffsetDateTime into a LocalDateTime of the Europe/Helsinki time zone.
*
* @param timestamp The value to convert.
* @return The converted value.
*/
public static LocalDateTime toLocalDateTimeFi(OffsetDateTime timestamp) {
return toLocalDateTime(timestamp, ZONE_HELSINKI);
}
/**
* Converts an OffsetDateTime into a LocalDateTime of the given time zone.
*
* @param timestamp The value to convert.
* @param zoneId The target time zone.
* @return The converted value.
*/
public static LocalDateTime toLocalDateTime(OffsetDateTime timestamp, ZoneId zoneId) {
if (timestamp == null)
return null;
return timestamp.atZoneSameInstant(zoneId).toLocalDateTime();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy