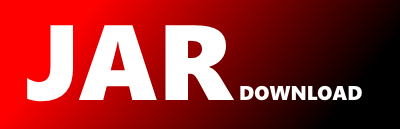
fi.evolver.utils.NullSafetyUtils Maven / Gradle / Ivy
package fi.evolver.utils;
import java.util.Comparator;
public class NullSafetyUtils {
private NullSafetyUtils() {
throw new IllegalStateException("Util class");
}
public static > int nullSafeCompare(T value1, T value2, boolean smallNull) {
if (value1 == null)
return value2 == null ? 0 : (smallNull ? -1 : 1);
if (value2 == null)
return smallNull ? 1 : -1;
return value1.compareTo(value2);
}
public static > int nullSafeCompare(T value1, T value2) {
return nullSafeCompare(value1, value2, true);
}
@SafeVarargs
public static T denull(T... values) {
for (T value: values) {
if (value != null)
return value;
}
return null;
}
/**
* Null safe comparator implementation.
* Tests arguments for null and calls argument comparator if both are non-null.
*
* @param The type to compare
*/
public static class NullSafeComparator implements Comparator {
private Comparator realComparator;
private boolean smallNull = true;
public NullSafeComparator(Comparator comparator) {
this.realComparator = comparator;
}
public NullSafeComparator(Comparator comparator, boolean smallNull) {
this.realComparator = comparator;
this.smallNull = smallNull;
}
@Override
public int compare(T o1, T o2) {
return nullCompare(o1, o2, realComparator, smallNull);
}
}
/**
* Null safe compareTo method. Calls nonNullComparator iff neither argument to compare is null.
*
* @param value1 The first value to compare
* @param value2 The second value to compare
* @param nonNullComparator The comparator to call in case both values are not null
* @return -1, 1 or 0
*/
public static int nullCompare(T value1, T value2, Comparator nonNullComparator) {
return nullCompare(value1, value2, nonNullComparator, true);
}
/**
* Null safe compareTo method. Calls nonNullComparator iff neither argument to compare is null.
*
* @param value1 The first value to compare
* @param value2 The second value to compare
* @param nonNullComparator The comparator to call in case both values are not null
* @param smallNull true if null is smaller than non-null
* @return -1, 1 or 0
*/
public static int nullCompare(T value1, T value2, Comparator nonNullComparator, boolean smallNull) {
if (value1 == null)
return value2 == null ? 0 : (smallNull ? -1 : 1);
if (value2 == null)
return smallNull ? 1 : -1;
return nonNullComparator.compare(value1, value2);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy