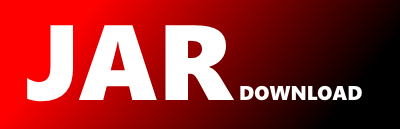
fi.evolver.utils.UriUtils Maven / Gradle / Ivy
package fi.evolver.utils;
import java.io.UnsupportedEncodingException;
import java.net.URI;
import java.net.URISyntaxException;
import java.net.URL;
import java.net.URLDecoder;
import java.net.URLEncoder;
import java.util.Optional;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class UriUtils {
public static Optional getQueryParameter(URI uri, String attributeName) {
return getParameter(uri.getRawQuery(), attributeName);
}
public static Optional getFragmentParameter(URI uri, String attributeName) {
return getParameter(uri.getRawFragment(), attributeName);
}
public static Optional getParameter(String data, String attributeName) {
if (data == null)
return Optional.empty();
Pattern pattern = Pattern.compile("(?:^|&)" + attributeName + "=([^&]*)");
Matcher matcher = pattern.matcher(data);
if (matcher.find()) {
try {
return Optional.of(URLDecoder.decode(matcher.group(1), "UTF-8"));
}
catch (UnsupportedEncodingException e) {
throw new IllegalStateException("BUG! UTF-8 not supported?", e);
}
}
return Optional.empty();
}
public static String getUserName(URL url) {
return parseUserInfo(url.getUserInfo())[0];
}
public static String getUserName(URI uri) {
return parseUserInfo(uri.getUserInfo())[0];
}
public static String getPassword(URL url) {
return parseUserInfo(url.getUserInfo())[1];
}
public static String getPassword(URI uri) {
return parseUserInfo(uri.getUserInfo())[1];
}
private static String[] parseUserInfo(String userInfo) {
if (userInfo == null)
return new String[2];
if (userInfo.contains(":"))
return userInfo.split(":", 2);
else
return new String[] { userInfo, null };
}
public static URI create(String protocol, String username, String password, String host, String path) throws URISyntaxException {
return create(protocol, username, password, host, path, null, null);
}
public static URI create(String protocol, String username, String password, String host, String path, String query, String fragment) throws URISyntaxException {
if (protocol == null || host == null || path == null)
throw new IllegalArgumentException(String.format("FAILED creating URI due to missing information. protocol:%s, host:%s, path:%s", protocol, host, path));
String userInfo = "";
if (username != null)
userInfo = username;
if (password != null)
userInfo += ':' + password;
int pivot = host.indexOf(':');
String hostName = host;
int port = -1;
if (pivot >= 0) {
port = Integer.parseInt(host.substring(pivot + 1));
hostName = host.substring(0, pivot);
}
return new URI(protocol, userInfo, hostName, port, path, query, fragment);
}
public static String encode(String value) {
try {
String result = URLEncoder.encode(value.replace("+", "PLUSSIGN"), "UTF-8");
return result.replace("+", "%20").replace("PLUSSIGN", "+");
}
catch (UnsupportedEncodingException e) {
return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy